Transaction Hash:
Block:
18228724 at Sep-27-2023 05:56:59 PM +UTC
Transaction Fee:
0.002294940814064149 ETH
$6.01
Gas Used:
88,243 Gas / 26.007057943 Gwei
Emitted Events:
243 |
NounsDAOProxy.VoteCast( voter=[Sender] 0x92a6f975cbb957677e44877008b4f85094350810, proposalId=143, support=1, votes=1, reason= )
|
244 |
NounsDAOProxy.0xfabef36fd46c4c3a6ad676521be5367a4dfdbf3faa68d8e826003b1752d68f4f( 0xfabef36fd46c4c3a6ad676521be5367a4dfdbf3faa68d8e826003b1752d68f4f, 0x00000000000000000000000092a6f975cbb957677e44877008b4f85094350810, 00000000000000000000000000000000000000000000000000082ca12440e691, 0000000000000000000000000000000000000000000000000000000000000001 )
|
Account State Difference:
Address | Before | After | State Difference | ||
---|---|---|---|---|---|
0x5d2C31ce...387854039 | 4.955396641082640117 Eth | 4.953095770659364964 Eth | 0.002300870423275153 | ||
0x92a6F975...094350810 |
0.170272976398019807 Eth
Nonce: 482
|
0.170278906007230811 Eth
Nonce: 483
| 0.000005929609211004 | ||
0xDAFEA492...692c98Bc5
Miner
| (Flashbots: Builder) | 1.545429101726750596 Eth | 1.545437926026750596 Eth | 0.0000088243 |
Execution Trace
NounsDAOProxy.44fac8f6( )
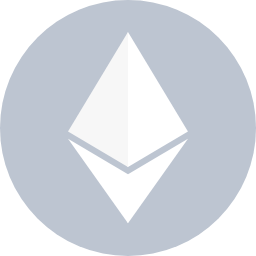
NounsDAOLogicV2.castRefundableVote( proposalId=143, support=1 )
-
NounsToken.getPriorVotes( account=0x92a6F975CBb957677E44877008b4F85094350810, blockNumber=18214187 ) => ( 1 )
- ETH 0.002300870423275153
0x92a6f975cbb957677e44877008b4f85094350810.CALL( )
-
File 1 of 3: NounsDAOProxy
File 2 of 3: NounsDAOLogicV2
File 3 of 3: NounsToken
// SPDX-License-Identifier: BSD-3-Clause /// @title The Nouns DAO proxy contract /********************************* * ░░░░░░░░░░░░░░░░░░░░░░░░░░░░░ * * ░░░░░░░░░░░░░░░░░░░░░░░░░░░░░ * * ░░░░░░█████████░░█████████░░░ * * ░░░░░░██░░░████░░██░░░████░░░ * * ░░██████░░░████████░░░████░░░ * * ░░██░░██░░░████░░██░░░████░░░ * * ░░██░░██░░░████░░██░░░████░░░ * * ░░░░░░█████████░░█████████░░░ * * ░░░░░░░░░░░░░░░░░░░░░░░░░░░░░ * * ░░░░░░░░░░░░░░░░░░░░░░░░░░░░░ * *********************************/ // LICENSE // NounsDAOProxy.sol is a modified version of Compound Lab's GovernorBravoDelegator.sol: // https://github.com/compound-finance/compound-protocol/blob/b9b14038612d846b83f8a009a82c38974ff2dcfe/contracts/Governance/GovernorBravoDelegator.sol // // GovernorBravoDelegator.sol source code Copyright 2020 Compound Labs, Inc. licensed under the BSD-3-Clause license. // With modifications by Nounders DAO. // // Additional conditions of BSD-3-Clause can be found here: https://opensource.org/licenses/BSD-3-Clause // // // NounsDAOProxy.sol uses parts of Open Zeppelin's Proxy.sol: // https://github.com/OpenZeppelin/openzeppelin-contracts/blob/5c8746f56b4bed8cc9e0e044f5f69ab2f9428ce1/contracts/proxy/Proxy.sol // // Proxy.sol source code licensed under MIT License. // // MODIFICATIONS // The fallback() and receive() functions of Proxy.sol have been used to allow Solidity > 0.6.0 compatibility pragma solidity ^0.8.6; import './NounsDAOInterfaces.sol'; contract NounsDAOProxy is NounsDAOProxyStorage, NounsDAOEvents { constructor( address timelock_, address nouns_, address vetoer_, address admin_, address implementation_, uint256 votingPeriod_, uint256 votingDelay_, uint256 proposalThresholdBPS_, uint256 quorumVotesBPS_ ) { // Admin set to msg.sender for initialization admin = msg.sender; delegateTo( implementation_, abi.encodeWithSignature( 'initialize(address,address,address,uint256,uint256,uint256,uint256)', timelock_, nouns_, vetoer_, votingPeriod_, votingDelay_, proposalThresholdBPS_, quorumVotesBPS_ ) ); _setImplementation(implementation_); admin = admin_; } /** * @notice Called by the admin to update the implementation of the delegator * @param implementation_ The address of the new implementation for delegation */ function _setImplementation(address implementation_) public { require(msg.sender == admin, 'NounsDAOProxy::_setImplementation: admin only'); require(implementation_ != address(0), 'NounsDAOProxy::_setImplementation: invalid implementation address'); address oldImplementation = implementation; implementation = implementation_; emit NewImplementation(oldImplementation, implementation); } /** * @notice Internal method to delegate execution to another contract * @dev It returns to the external caller whatever the implementation returns or forwards reverts * @param callee The contract to delegatecall * @param data The raw data to delegatecall */ function delegateTo(address callee, bytes memory data) internal { (bool success, bytes memory returnData) = callee.delegatecall(data); assembly { if eq(success, 0) { revert(add(returnData, 0x20), returndatasize()) } } } /** * @dev Delegates execution to an implementation contract. * It returns to the external caller whatever the implementation returns * or forwards reverts. */ function _fallback() internal { // delegate all other functions to current implementation (bool success, ) = implementation.delegatecall(msg.data); assembly { let free_mem_ptr := mload(0x40) returndatacopy(free_mem_ptr, 0, returndatasize()) switch success case 0 { revert(free_mem_ptr, returndatasize()) } default { return(free_mem_ptr, returndatasize()) } } } /** * @dev Fallback function that delegates calls to the `implementation`. Will run if no other * function in the contract matches the call data. */ fallback() external payable { _fallback(); } /** * @dev Fallback function that delegates calls to `implementation`. Will run if call data * is empty. */ receive() external payable { _fallback(); } } // SPDX-License-Identifier: BSD-3-Clause /// @title Nouns DAO Logic interfaces and events /********************************* * ░░░░░░░░░░░░░░░░░░░░░░░░░░░░░ * * ░░░░░░░░░░░░░░░░░░░░░░░░░░░░░ * * ░░░░░░█████████░░█████████░░░ * * ░░░░░░██░░░████░░██░░░████░░░ * * ░░██████░░░████████░░░████░░░ * * ░░██░░██░░░████░░██░░░████░░░ * * ░░██░░██░░░████░░██░░░████░░░ * * ░░░░░░█████████░░█████████░░░ * * ░░░░░░░░░░░░░░░░░░░░░░░░░░░░░ * * ░░░░░░░░░░░░░░░░░░░░░░░░░░░░░ * *********************************/ // LICENSE // NounsDAOInterfaces.sol is a modified version of Compound Lab's GovernorBravoInterfaces.sol: // https://github.com/compound-finance/compound-protocol/blob/b9b14038612d846b83f8a009a82c38974ff2dcfe/contracts/Governance/GovernorBravoInterfaces.sol // // GovernorBravoInterfaces.sol source code Copyright 2020 Compound Labs, Inc. licensed under the BSD-3-Clause license. // With modifications by Nounders DAO. // // Additional conditions of BSD-3-Clause can be found here: https://opensource.org/licenses/BSD-3-Clause // // MODIFICATIONS // NounsDAOEvents, NounsDAOProxyStorage, NounsDAOStorageV1 adds support for changes made by Nouns DAO to GovernorBravo.sol // See NounsDAOLogicV1.sol for more details. pragma solidity ^0.8.6; contract NounsDAOEvents { /// @notice An event emitted when a new proposal is created event ProposalCreated( uint256 id, address proposer, address[] targets, uint256[] values, string[] signatures, bytes[] calldatas, uint256 startBlock, uint256 endBlock, string description ); event ProposalCreatedWithRequirements( uint256 id, address proposer, address[] targets, uint256[] values, string[] signatures, bytes[] calldatas, uint256 startBlock, uint256 endBlock, uint256 proposalThreshold, uint256 quorumVotes, string description ); /// @notice An event emitted when a vote has been cast on a proposal /// @param voter The address which casted a vote /// @param proposalId The proposal id which was voted on /// @param support Support value for the vote. 0=against, 1=for, 2=abstain /// @param votes Number of votes which were cast by the voter /// @param reason The reason given for the vote by the voter event VoteCast(address indexed voter, uint256 proposalId, uint8 support, uint256 votes, string reason); /// @notice An event emitted when a proposal has been canceled event ProposalCanceled(uint256 id); /// @notice An event emitted when a proposal has been queued in the NounsDAOExecutor event ProposalQueued(uint256 id, uint256 eta); /// @notice An event emitted when a proposal has been executed in the NounsDAOExecutor event ProposalExecuted(uint256 id); /// @notice An event emitted when a proposal has been vetoed by vetoAddress event ProposalVetoed(uint256 id); /// @notice An event emitted when the voting delay is set event VotingDelaySet(uint256 oldVotingDelay, uint256 newVotingDelay); /// @notice An event emitted when the voting period is set event VotingPeriodSet(uint256 oldVotingPeriod, uint256 newVotingPeriod); /// @notice Emitted when implementation is changed event NewImplementation(address oldImplementation, address newImplementation); /// @notice Emitted when proposal threshold basis points is set event ProposalThresholdBPSSet(uint256 oldProposalThresholdBPS, uint256 newProposalThresholdBPS); /// @notice Emitted when quorum votes basis points is set event QuorumVotesBPSSet(uint256 oldQuorumVotesBPS, uint256 newQuorumVotesBPS); /// @notice Emitted when pendingAdmin is changed event NewPendingAdmin(address oldPendingAdmin, address newPendingAdmin); /// @notice Emitted when pendingAdmin is accepted, which means admin is updated event NewAdmin(address oldAdmin, address newAdmin); /// @notice Emitted when vetoer is changed event NewVetoer(address oldVetoer, address newVetoer); } contract NounsDAOProxyStorage { /// @notice Administrator for this contract address public admin; /// @notice Pending administrator for this contract address public pendingAdmin; /// @notice Active brains of Governor address public implementation; } /** * @title Storage for Governor Bravo Delegate * @notice For future upgrades, do not change NounsDAOStorageV1. Create a new * contract which implements NounsDAOStorageV1 and following the naming convention * NounsDAOStorageVX. */ contract NounsDAOStorageV1 is NounsDAOProxyStorage { /// @notice Vetoer who has the ability to veto any proposal address public vetoer; /// @notice The delay before voting on a proposal may take place, once proposed, in blocks uint256 public votingDelay; /// @notice The duration of voting on a proposal, in blocks uint256 public votingPeriod; /// @notice The basis point number of votes required in order for a voter to become a proposer. *DIFFERS from GovernerBravo uint256 public proposalThresholdBPS; /// @notice The basis point number of votes in support of a proposal required in order for a quorum to be reached and for a vote to succeed. *DIFFERS from GovernerBravo uint256 public quorumVotesBPS; /// @notice The total number of proposals uint256 public proposalCount; /// @notice The address of the Nouns DAO Executor NounsDAOExecutor INounsDAOExecutor public timelock; /// @notice The address of the Nouns tokens NounsTokenLike public nouns; /// @notice The official record of all proposals ever proposed mapping(uint256 => Proposal) public proposals; /// @notice The latest proposal for each proposer mapping(address => uint256) public latestProposalIds; struct Proposal { /// @notice Unique id for looking up a proposal uint256 id; /// @notice Creator of the proposal address proposer; /// @notice The number of votes needed to create a proposal at the time of proposal creation. *DIFFERS from GovernerBravo uint256 proposalThreshold; /// @notice The number of votes in support of a proposal required in order for a quorum to be reached and for a vote to succeed at the time of proposal creation. *DIFFERS from GovernerBravo uint256 quorumVotes; /// @notice The timestamp that the proposal will be available for execution, set once the vote succeeds uint256 eta; /// @notice the ordered list of target addresses for calls to be made address[] targets; /// @notice The ordered list of values (i.e. msg.value) to be passed to the calls to be made uint256[] values; /// @notice The ordered list of function signatures to be called string[] signatures; /// @notice The ordered list of calldata to be passed to each call bytes[] calldatas; /// @notice The block at which voting begins: holders must delegate their votes prior to this block uint256 startBlock; /// @notice The block at which voting ends: votes must be cast prior to this block uint256 endBlock; /// @notice Current number of votes in favor of this proposal uint256 forVotes; /// @notice Current number of votes in opposition to this proposal uint256 againstVotes; /// @notice Current number of votes for abstaining for this proposal uint256 abstainVotes; /// @notice Flag marking whether the proposal has been canceled bool canceled; /// @notice Flag marking whether the proposal has been vetoed bool vetoed; /// @notice Flag marking whether the proposal has been executed bool executed; /// @notice Receipts of ballots for the entire set of voters mapping(address => Receipt) receipts; } /// @notice Ballot receipt record for a voter struct Receipt { /// @notice Whether or not a vote has been cast bool hasVoted; /// @notice Whether or not the voter supports the proposal or abstains uint8 support; /// @notice The number of votes the voter had, which were cast uint96 votes; } /// @notice Possible states that a proposal may be in enum ProposalState { Pending, Active, Canceled, Defeated, Succeeded, Queued, Expired, Executed, Vetoed } } interface INounsDAOExecutor { function delay() external view returns (uint256); function GRACE_PERIOD() external view returns (uint256); function acceptAdmin() external; function queuedTransactions(bytes32 hash) external view returns (bool); function queueTransaction( address target, uint256 value, string calldata signature, bytes calldata data, uint256 eta ) external returns (bytes32); function cancelTransaction( address target, uint256 value, string calldata signature, bytes calldata data, uint256 eta ) external; function executeTransaction( address target, uint256 value, string calldata signature, bytes calldata data, uint256 eta ) external payable returns (bytes memory); } interface NounsTokenLike { function getPriorVotes(address account, uint256 blockNumber) external view returns (uint96); function totalSupply() external view returns (uint96); }
File 2 of 3: NounsDAOLogicV2
{"NounsDAOInterfaces.sol":{"content":"// SPDX-License-Identifier: BSD-3-Clause\n\n/// @title Nouns DAO Logic interfaces and events\n\n/*********************************\n * ░░░░░░░░░░░░░░░░░░░░░░░░░░░░░ *\n * ░░░░░░░░░░░░░░░░░░░░░░░░░░░░░ *\n * ░░░░░░█████████░░█████████░░░ *\n * ░░░░░░██░░░████░░██░░░████░░░ *\n * ░░██████░░░████████░░░████░░░ *\n * ░░██░░██░░░████░░██░░░████░░░ *\n * ░░██░░██░░░████░░██░░░████░░░ *\n * ░░░░░░█████████░░█████████░░░ *\n * ░░░░░░░░░░░░░░░░░░░░░░░░░░░░░ *\n * ░░░░░░░░░░░░░░░░░░░░░░░░░░░░░ *\n *********************************/\n\n// LICENSE\n// NounsDAOInterfaces.sol is a modified version of Compound Lab\u0027s GovernorBravoInterfaces.sol:\n// https://github.com/compound-finance/compound-protocol/blob/b9b14038612d846b83f8a009a82c38974ff2dcfe/contracts/Governance/GovernorBravoInterfaces.sol\n//\n// GovernorBravoInterfaces.sol source code Copyright 2020 Compound Labs, Inc. licensed under the BSD-3-Clause license.\n// With modifications by Nounders DAO.\n//\n// Additional conditions of BSD-3-Clause can be found here: https://opensource.org/licenses/BSD-3-Clause\n//\n// MODIFICATIONS\n// NounsDAOEvents, NounsDAOProxyStorage, NounsDAOStorageV1 add support for changes made by Nouns DAO to GovernorBravo.sol\n// See NounsDAOLogicV1.sol for more details.\n// NounsDAOStorageV1Adjusted and NounsDAOStorageV2 add support for a dynamic vote quorum.\n// See NounsDAOLogicV2.sol for more details.\n\npragma solidity ^0.8.6;\n\ncontract NounsDAOEvents {\n /// @notice An event emitted when a new proposal is created\n event ProposalCreated(\n uint256 id,\n address proposer,\n address[] targets,\n uint256[] values,\n string[] signatures,\n bytes[] calldatas,\n uint256 startBlock,\n uint256 endBlock,\n string description\n );\n\n /// @notice An event emitted when a new proposal is created, which includes additional information\n event ProposalCreatedWithRequirements(\n uint256 id,\n address proposer,\n address[] targets,\n uint256[] values,\n string[] signatures,\n bytes[] calldatas,\n uint256 startBlock,\n uint256 endBlock,\n uint256 proposalThreshold,\n uint256 quorumVotes,\n string description\n );\n\n /// @notice An event emitted when a vote has been cast on a proposal\n /// @param voter The address which casted a vote\n /// @param proposalId The proposal id which was voted on\n /// @param support Support value for the vote. 0=against, 1=for, 2=abstain\n /// @param votes Number of votes which were cast by the voter\n /// @param reason The reason given for the vote by the voter\n event VoteCast(address indexed voter, uint256 proposalId, uint8 support, uint256 votes, string reason);\n\n /// @notice An event emitted when a proposal has been canceled\n event ProposalCanceled(uint256 id);\n\n /// @notice An event emitted when a proposal has been queued in the NounsDAOExecutor\n event ProposalQueued(uint256 id, uint256 eta);\n\n /// @notice An event emitted when a proposal has been executed in the NounsDAOExecutor\n event ProposalExecuted(uint256 id);\n\n /// @notice An event emitted when a proposal has been vetoed by vetoAddress\n event ProposalVetoed(uint256 id);\n\n /// @notice An event emitted when the voting delay is set\n event VotingDelaySet(uint256 oldVotingDelay, uint256 newVotingDelay);\n\n /// @notice An event emitted when the voting period is set\n event VotingPeriodSet(uint256 oldVotingPeriod, uint256 newVotingPeriod);\n\n /// @notice Emitted when implementation is changed\n event NewImplementation(address oldImplementation, address newImplementation);\n\n /// @notice Emitted when proposal threshold basis points is set\n event ProposalThresholdBPSSet(uint256 oldProposalThresholdBPS, uint256 newProposalThresholdBPS);\n\n /// @notice Emitted when quorum votes basis points is set\n event QuorumVotesBPSSet(uint256 oldQuorumVotesBPS, uint256 newQuorumVotesBPS);\n\n /// @notice Emitted when pendingAdmin is changed\n event NewPendingAdmin(address oldPendingAdmin, address newPendingAdmin);\n\n /// @notice Emitted when pendingAdmin is accepted, which means admin is updated\n event NewAdmin(address oldAdmin, address newAdmin);\n\n /// @notice Emitted when vetoer is changed\n event NewVetoer(address oldVetoer, address newVetoer);\n}\n\ncontract NounsDAOEventsV2 is NounsDAOEvents {\n /// @notice Emitted when minQuorumVotesBPS is set\n event MinQuorumVotesBPSSet(uint16 oldMinQuorumVotesBPS, uint16 newMinQuorumVotesBPS);\n\n /// @notice Emitted when maxQuorumVotesBPS is set\n event MaxQuorumVotesBPSSet(uint16 oldMaxQuorumVotesBPS, uint16 newMaxQuorumVotesBPS);\n\n /// @notice Emitted when quorumCoefficient is set\n event QuorumCoefficientSet(uint32 oldQuorumCoefficient, uint32 newQuorumCoefficient);\n\n /// @notice Emitted when a voter cast a vote requesting a gas refund.\n event RefundableVote(address indexed voter, uint256 refundAmount, bool refundSent);\n\n /// @notice Emitted when admin withdraws the DAO\u0027s balance.\n event Withdraw(uint256 amount, bool sent);\n\n /// @notice Emitted when pendingVetoer is changed\n event NewPendingVetoer(address oldPendingVetoer, address newPendingVetoer);\n}\n\ncontract NounsDAOProxyStorage {\n /// @notice Administrator for this contract\n address public admin;\n\n /// @notice Pending administrator for this contract\n address public pendingAdmin;\n\n /// @notice Active brains of Governor\n address public implementation;\n}\n\n/**\n * @title Storage for Governor Bravo Delegate\n * @notice For future upgrades, do not change NounsDAOStorageV1. Create a new\n * contract which implements NounsDAOStorageV1 and following the naming convention\n * NounsDAOStorageVX.\n */\ncontract NounsDAOStorageV1 is NounsDAOProxyStorage {\n /// @notice Vetoer who has the ability to veto any proposal\n address public vetoer;\n\n /// @notice The delay before voting on a proposal may take place, once proposed, in blocks\n uint256 public votingDelay;\n\n /// @notice The duration of voting on a proposal, in blocks\n uint256 public votingPeriod;\n\n /// @notice The basis point number of votes required in order for a voter to become a proposer. *DIFFERS from GovernerBravo\n uint256 public proposalThresholdBPS;\n\n /// @notice The basis point number of votes in support of a proposal required in order for a quorum to be reached and for a vote to succeed. *DIFFERS from GovernerBravo\n uint256 public quorumVotesBPS;\n\n /// @notice The total number of proposals\n uint256 public proposalCount;\n\n /// @notice The address of the Nouns DAO Executor NounsDAOExecutor\n INounsDAOExecutor public timelock;\n\n /// @notice The address of the Nouns tokens\n NounsTokenLike public nouns;\n\n /// @notice The official record of all proposals ever proposed\n mapping(uint256 =\u003e Proposal) public proposals;\n\n /// @notice The latest proposal for each proposer\n mapping(address =\u003e uint256) public latestProposalIds;\n\n struct Proposal {\n /// @notice Unique id for looking up a proposal\n uint256 id;\n /// @notice Creator of the proposal\n address proposer;\n /// @notice The number of votes needed to create a proposal at the time of proposal creation. *DIFFERS from GovernerBravo\n uint256 proposalThreshold;\n /// @notice The number of votes in support of a proposal required in order for a quorum to be reached and for a vote to succeed at the time of proposal creation. *DIFFERS from GovernerBravo\n uint256 quorumVotes;\n /// @notice The timestamp that the proposal will be available for execution, set once the vote succeeds\n uint256 eta;\n /// @notice the ordered list of target addresses for calls to be made\n address[] targets;\n /// @notice The ordered list of values (i.e. msg.value) to be passed to the calls to be made\n uint256[] values;\n /// @notice The ordered list of function signatures to be called\n string[] signatures;\n /// @notice The ordered list of calldata to be passed to each call\n bytes[] calldatas;\n /// @notice The block at which voting begins: holders must delegate their votes prior to this block\n uint256 startBlock;\n /// @notice The block at which voting ends: votes must be cast prior to this block\n uint256 endBlock;\n /// @notice Current number of votes in favor of this proposal\n uint256 forVotes;\n /// @notice Current number of votes in opposition to this proposal\n uint256 againstVotes;\n /// @notice Current number of votes for abstaining for this proposal\n uint256 abstainVotes;\n /// @notice Flag marking whether the proposal has been canceled\n bool canceled;\n /// @notice Flag marking whether the proposal has been vetoed\n bool vetoed;\n /// @notice Flag marking whether the proposal has been executed\n bool executed;\n /// @notice Receipts of ballots for the entire set of voters\n mapping(address =\u003e Receipt) receipts;\n }\n\n /// @notice Ballot receipt record for a voter\n struct Receipt {\n /// @notice Whether or not a vote has been cast\n bool hasVoted;\n /// @notice Whether or not the voter supports the proposal or abstains\n uint8 support;\n /// @notice The number of votes the voter had, which were cast\n uint96 votes;\n }\n\n /// @notice Possible states that a proposal may be in\n enum ProposalState {\n Pending,\n Active,\n Canceled,\n Defeated,\n Succeeded,\n Queued,\n Expired,\n Executed,\n Vetoed\n }\n}\n\n/**\n * @title Extra fields added to the `Proposal` struct from NounsDAOStorageV1\n * @notice The following fields were added to the `Proposal` struct:\n * - `Proposal.totalSupply`\n * - `Proposal.creationBlock`\n */\ncontract NounsDAOStorageV1Adjusted is NounsDAOProxyStorage {\n /// @notice Vetoer who has the ability to veto any proposal\n address public vetoer;\n\n /// @notice The delay before voting on a proposal may take place, once proposed, in blocks\n uint256 public votingDelay;\n\n /// @notice The duration of voting on a proposal, in blocks\n uint256 public votingPeriod;\n\n /// @notice The basis point number of votes required in order for a voter to become a proposer. *DIFFERS from GovernerBravo\n uint256 public proposalThresholdBPS;\n\n /// @notice The basis point number of votes in support of a proposal required in order for a quorum to be reached and for a vote to succeed. *DIFFERS from GovernerBravo\n uint256 public quorumVotesBPS;\n\n /// @notice The total number of proposals\n uint256 public proposalCount;\n\n /// @notice The address of the Nouns DAO Executor NounsDAOExecutor\n INounsDAOExecutor public timelock;\n\n /// @notice The address of the Nouns tokens\n NounsTokenLike public nouns;\n\n /// @notice The official record of all proposals ever proposed\n mapping(uint256 =\u003e Proposal) internal _proposals;\n\n /// @notice The latest proposal for each proposer\n mapping(address =\u003e uint256) public latestProposalIds;\n\n struct Proposal {\n /// @notice Unique id for looking up a proposal\n uint256 id;\n /// @notice Creator of the proposal\n address proposer;\n /// @notice The number of votes needed to create a proposal at the time of proposal creation. *DIFFERS from GovernerBravo\n uint256 proposalThreshold;\n /// @notice The number of votes in support of a proposal required in order for a quorum to be reached and for a vote to succeed at the time of proposal creation. *DIFFERS from GovernerBravo\n uint256 quorumVotes;\n /// @notice The timestamp that the proposal will be available for execution, set once the vote succeeds\n uint256 eta;\n /// @notice the ordered list of target addresses for calls to be made\n address[] targets;\n /// @notice The ordered list of values (i.e. msg.value) to be passed to the calls to be made\n uint256[] values;\n /// @notice The ordered list of function signatures to be called\n string[] signatures;\n /// @notice The ordered list of calldata to be passed to each call\n bytes[] calldatas;\n /// @notice The block at which voting begins: holders must delegate their votes prior to this block\n uint256 startBlock;\n /// @notice The block at which voting ends: votes must be cast prior to this block\n uint256 endBlock;\n /// @notice Current number of votes in favor of this proposal\n uint256 forVotes;\n /// @notice Current number of votes in opposition to this proposal\n uint256 againstVotes;\n /// @notice Current number of votes for abstaining for this proposal\n uint256 abstainVotes;\n /// @notice Flag marking whether the proposal has been canceled\n bool canceled;\n /// @notice Flag marking whether the proposal has been vetoed\n bool vetoed;\n /// @notice Flag marking whether the proposal has been executed\n bool executed;\n /// @notice Receipts of ballots for the entire set of voters\n mapping(address =\u003e Receipt) receipts;\n /// @notice The total supply at the time of proposal creation\n uint256 totalSupply;\n /// @notice The block at which this proposal was created\n uint256 creationBlock;\n }\n\n /// @notice Ballot receipt record for a voter\n struct Receipt {\n /// @notice Whether or not a vote has been cast\n bool hasVoted;\n /// @notice Whether or not the voter supports the proposal or abstains\n uint8 support;\n /// @notice The number of votes the voter had, which were cast\n uint96 votes;\n }\n\n /// @notice Possible states that a proposal may be in\n enum ProposalState {\n Pending,\n Active,\n Canceled,\n Defeated,\n Succeeded,\n Queued,\n Expired,\n Executed,\n Vetoed\n }\n}\n\n/**\n * @title Storage for Governor Bravo Delegate\n * @notice For future upgrades, do not change NounsDAOStorageV2. Create a new\n * contract which implements NounsDAOStorageV2 and following the naming convention\n * NounsDAOStorageVX.\n */\ncontract NounsDAOStorageV2 is NounsDAOStorageV1Adjusted {\n DynamicQuorumParamsCheckpoint[] public quorumParamsCheckpoints;\n\n /// @notice Pending new vetoer\n address public pendingVetoer;\n\n struct DynamicQuorumParams {\n /// @notice The minimum basis point number of votes in support of a proposal required in order for a quorum to be reached and for a vote to succeed.\n uint16 minQuorumVotesBPS;\n /// @notice The maximum basis point number of votes in support of a proposal required in order for a quorum to be reached and for a vote to succeed.\n uint16 maxQuorumVotesBPS;\n /// @notice The dynamic quorum coefficient\n /// @dev Assumed to be fixed point integer with 6 decimals, i.e 0.2 is represented as 0.2 * 1e6 = 200000\n uint32 quorumCoefficient;\n }\n\n /// @notice A checkpoint for storing dynamic quorum params from a given block\n struct DynamicQuorumParamsCheckpoint {\n /// @notice The block at which the new values were set\n uint32 fromBlock;\n /// @notice The parameter values of this checkpoint\n DynamicQuorumParams params;\n }\n\n struct ProposalCondensed {\n /// @notice Unique id for looking up a proposal\n uint256 id;\n /// @notice Creator of the proposal\n address proposer;\n /// @notice The number of votes needed to create a proposal at the time of proposal creation. *DIFFERS from GovernerBravo\n uint256 proposalThreshold;\n /// @notice The minimum number of votes in support of a proposal required in order for a quorum to be reached and for a vote to succeed at the time of proposal creation. *DIFFERS from GovernerBravo\n uint256 quorumVotes;\n /// @notice The timestamp that the proposal will be available for execution, set once the vote succeeds\n uint256 eta;\n /// @notice The block at which voting begins: holders must delegate their votes prior to this block\n uint256 startBlock;\n /// @notice The block at which voting ends: votes must be cast prior to this block\n uint256 endBlock;\n /// @notice Current number of votes in favor of this proposal\n uint256 forVotes;\n /// @notice Current number of votes in opposition to this proposal\n uint256 againstVotes;\n /// @notice Current number of votes for abstaining for this proposal\n uint256 abstainVotes;\n /// @notice Flag marking whether the proposal has been canceled\n bool canceled;\n /// @notice Flag marking whether the proposal has been vetoed\n bool vetoed;\n /// @notice Flag marking whether the proposal has been executed\n bool executed;\n /// @notice The total supply at the time of proposal creation\n uint256 totalSupply;\n /// @notice The block at which this proposal was created\n uint256 creationBlock;\n }\n}\n\ninterface INounsDAOExecutor {\n function delay() external view returns (uint256);\n\n function GRACE_PERIOD() external view returns (uint256);\n\n function acceptAdmin() external;\n\n function queuedTransactions(bytes32 hash) external view returns (bool);\n\n function queueTransaction(\n address target,\n uint256 value,\n string calldata signature,\n bytes calldata data,\n uint256 eta\n ) external returns (bytes32);\n\n function cancelTransaction(\n address target,\n uint256 value,\n string calldata signature,\n bytes calldata data,\n uint256 eta\n ) external;\n\n function executeTransaction(\n address target,\n uint256 value,\n string calldata signature,\n bytes calldata data,\n uint256 eta\n ) external payable returns (bytes memory);\n}\n\ninterface NounsTokenLike {\n function getPriorVotes(address account, uint256 blockNumber) external view returns (uint96);\n\n function totalSupply() external view returns (uint256);\n}\n"},"NounsDAOLogicV2.sol":{"content":"// SPDX-License-Identifier: BSD-3-Clause\n\n/// @title The Nouns DAO logic version 2\n\n/*********************************\n * ░░░░░░░░░░░░░░░░░░░░░░░░░░░░░ *\n * ░░░░░░░░░░░░░░░░░░░░░░░░░░░░░ *\n * ░░░░░░█████████░░█████████░░░ *\n * ░░░░░░██░░░████░░██░░░████░░░ *\n * ░░██████░░░████████░░░████░░░ *\n * ░░██░░██░░░████░░██░░░████░░░ *\n * ░░██░░██░░░████░░██░░░████░░░ *\n * ░░░░░░█████████░░█████████░░░ *\n * ░░░░░░░░░░░░░░░░░░░░░░░░░░░░░ *\n * ░░░░░░░░░░░░░░░░░░░░░░░░░░░░░ *\n *********************************/\n\n// LICENSE\n// NounsDAOLogicV2.sol is a modified version of Compound Lab\u0027s GovernorBravoDelegate.sol:\n// https://github.com/compound-finance/compound-protocol/blob/b9b14038612d846b83f8a009a82c38974ff2dcfe/contracts/Governance/GovernorBravoDelegate.sol\n//\n// GovernorBravoDelegate.sol source code Copyright 2020 Compound Labs, Inc. licensed under the BSD-3-Clause license.\n// With modifications by Nounders DAO.\n//\n// Additional conditions of BSD-3-Clause can be found here: https://opensource.org/licenses/BSD-3-Clause\n//\n// MODIFICATIONS\n// See NounsDAOLogicV1 for initial GovernorBravoDelegate modifications.\n\n// NounsDAOLogicV2 adds:\n// - `quorumParamsCheckpoints`, which store dynamic quorum parameters checkpoints\n// to be used when calculating the dynamic quorum.\n// - `_setDynamicQuorumParams(DynamicQuorumParams memory params)`, which allows the\n// DAO to update the dynamic quorum parameters\u0027 values.\n// - `getDynamicQuorumParamsAt(uint256 blockNumber_)`\n// - Individual setters of the DynamicQuorumParams members:\n// - `_setMinQuorumVotesBPS(uint16 newMinQuorumVotesBPS)`\n// - `_setMaxQuorumVotesBPS(uint16 newMaxQuorumVotesBPS)`\n// - `_setQuorumCoefficient(uint32 newQuorumCoefficient)`\n// - `minQuorumVotes` and `maxQuorumVotes`, which returns the current min and\n// max quorum votes using the current Noun supply.\n// - New `Proposal` struct member:\n// - `totalSupply` used in dynamic quorum calculation.\n// - `creationBlock` used for retrieving checkpoints of votes and dynamic quorum params. This now\n// allows changing `votingDelay` without affecting the checkpoints lookup.\n// - `quorumVotes(uint256 proposalId)`, which calculates and returns the dynamic\n// quorum for a specific proposal.\n// - `proposals(uint256 proposalId)` instead of the implicit getter, to avoid stack-too-deep error\n//\n// NounsDAOLogicV2 removes:\n// - `quorumVotes()` has been replaced by `quorumVotes(uint256 proposalId)`.\n\npragma solidity ^0.8.6;\n\nimport \u0027./NounsDAOInterfaces.sol\u0027;\n\ncontract NounsDAOLogicV2 is NounsDAOStorageV2, NounsDAOEventsV2 {\n /// @notice The name of this contract\n string public constant name = \u0027Nouns DAO\u0027;\n\n /// @notice The minimum setable proposal threshold\n uint256 public constant MIN_PROPOSAL_THRESHOLD_BPS = 1; // 1 basis point or 0.01%\n\n /// @notice The maximum setable proposal threshold\n uint256 public constant MAX_PROPOSAL_THRESHOLD_BPS = 1_000; // 1,000 basis points or 10%\n\n /// @notice The minimum setable voting period\n uint256 public constant MIN_VOTING_PERIOD = 5_760; // About 24 hours\n\n /// @notice The max setable voting period\n uint256 public constant MAX_VOTING_PERIOD = 80_640; // About 2 weeks\n\n /// @notice The min setable voting delay\n uint256 public constant MIN_VOTING_DELAY = 1;\n\n /// @notice The max setable voting delay\n uint256 public constant MAX_VOTING_DELAY = 40_320; // About 1 week\n\n /// @notice The lower bound of minimum quorum votes basis points\n uint256 public constant MIN_QUORUM_VOTES_BPS_LOWER_BOUND = 200; // 200 basis points or 2%\n\n /// @notice The upper bound of minimum quorum votes basis points\n uint256 public constant MIN_QUORUM_VOTES_BPS_UPPER_BOUND = 2_000; // 2,000 basis points or 20%\n\n /// @notice The upper bound of maximum quorum votes basis points\n uint256 public constant MAX_QUORUM_VOTES_BPS_UPPER_BOUND = 6_000; // 4,000 basis points or 60%\n\n /// @notice The maximum setable quorum votes basis points\n uint256 public constant MAX_QUORUM_VOTES_BPS = 2_000; // 2,000 basis points or 20%\n\n /// @notice The maximum number of actions that can be included in a proposal\n uint256 public constant proposalMaxOperations = 10; // 10 actions\n\n /// @notice The maximum priority fee used to cap gas refunds in `castRefundableVote`\n uint256 public constant MAX_REFUND_PRIORITY_FEE = 2 gwei;\n\n /// @notice The vote refund gas overhead, including 7K for ETH transfer and 29K for general transaction overhead\n uint256 public constant REFUND_BASE_GAS = 36000;\n\n /// @notice The maximum gas units the DAO will refund voters on; supports about 9,190 characters\n uint256 public constant MAX_REFUND_GAS_USED = 200_000;\n\n /// @notice The maximum basefee the DAO will refund voters on\n uint256 public constant MAX_REFUND_BASE_FEE = 200 gwei;\n\n /// @notice The EIP-712 typehash for the contract\u0027s domain\n bytes32 public constant DOMAIN_TYPEHASH =\n keccak256(\u0027EIP712Domain(string name,uint256 chainId,address verifyingContract)\u0027);\n\n /// @notice The EIP-712 typehash for the ballot struct used by the contract\n bytes32 public constant BALLOT_TYPEHASH = keccak256(\u0027Ballot(uint256 proposalId,uint8 support)\u0027);\n\n /// @dev Introduced these errors to reduce contract size, to avoid deployment failure\n error AdminOnly();\n error InvalidMinQuorumVotesBPS();\n error InvalidMaxQuorumVotesBPS();\n error MinQuorumBPSGreaterThanMaxQuorumBPS();\n error UnsafeUint16Cast();\n error VetoerOnly();\n error PendingVetoerOnly();\n error VetoerBurned();\n error CantVetoExecutedProposal();\n error CantCancelExecutedProposal();\n\n /**\n * @notice Used to initialize the contract during delegator contructor\n * @param timelock_ The address of the NounsDAOExecutor\n * @param nouns_ The address of the NOUN tokens\n * @param vetoer_ The address allowed to unilaterally veto proposals\n * @param votingPeriod_ The initial voting period\n * @param votingDelay_ The initial voting delay\n * @param proposalThresholdBPS_ The initial proposal threshold in basis points\n * @param dynamicQuorumParams_ The initial dynamic quorum parameters\n */\n function initialize(\n address timelock_,\n address nouns_,\n address vetoer_,\n uint256 votingPeriod_,\n uint256 votingDelay_,\n uint256 proposalThresholdBPS_,\n DynamicQuorumParams calldata dynamicQuorumParams_\n ) public virtual {\n require(address(timelock) == address(0), \u0027NounsDAO::initialize: can only initialize once\u0027);\n if (msg.sender != admin) {\n revert AdminOnly();\n }\n require(timelock_ != address(0), \u0027NounsDAO::initialize: invalid timelock address\u0027);\n require(nouns_ != address(0), \u0027NounsDAO::initialize: invalid nouns address\u0027);\n require(\n votingPeriod_ \u003e= MIN_VOTING_PERIOD \u0026\u0026 votingPeriod_ \u003c= MAX_VOTING_PERIOD,\n \u0027NounsDAO::initialize: invalid voting period\u0027\n );\n require(\n votingDelay_ \u003e= MIN_VOTING_DELAY \u0026\u0026 votingDelay_ \u003c= MAX_VOTING_DELAY,\n \u0027NounsDAO::initialize: invalid voting delay\u0027\n );\n require(\n proposalThresholdBPS_ \u003e= MIN_PROPOSAL_THRESHOLD_BPS \u0026\u0026 proposalThresholdBPS_ \u003c= MAX_PROPOSAL_THRESHOLD_BPS,\n \u0027NounsDAO::initialize: invalid proposal threshold bps\u0027\n );\n\n emit VotingPeriodSet(votingPeriod, votingPeriod_);\n emit VotingDelaySet(votingDelay, votingDelay_);\n emit ProposalThresholdBPSSet(proposalThresholdBPS, proposalThresholdBPS_);\n\n timelock = INounsDAOExecutor(timelock_);\n nouns = NounsTokenLike(nouns_);\n vetoer = vetoer_;\n votingPeriod = votingPeriod_;\n votingDelay = votingDelay_;\n proposalThresholdBPS = proposalThresholdBPS_;\n _setDynamicQuorumParams(\n dynamicQuorumParams_.minQuorumVotesBPS,\n dynamicQuorumParams_.maxQuorumVotesBPS,\n dynamicQuorumParams_.quorumCoefficient\n );\n }\n\n struct ProposalTemp {\n uint256 totalSupply;\n uint256 proposalThreshold;\n uint256 latestProposalId;\n uint256 startBlock;\n uint256 endBlock;\n }\n\n /**\n * @notice Function used to propose a new proposal. Sender must have delegates above the proposal threshold\n * @param targets Target addresses for proposal calls\n * @param values Eth values for proposal calls\n * @param signatures Function signatures for proposal calls\n * @param calldatas Calldatas for proposal calls\n * @param description String description of the proposal\n * @return Proposal id of new proposal\n */\n function propose(\n address[] memory targets,\n uint256[] memory values,\n string[] memory signatures,\n bytes[] memory calldatas,\n string memory description\n ) public returns (uint256) {\n ProposalTemp memory temp;\n\n temp.totalSupply = nouns.totalSupply();\n\n temp.proposalThreshold = bps2Uint(proposalThresholdBPS, temp.totalSupply);\n\n require(\n nouns.getPriorVotes(msg.sender, block.number - 1) \u003e temp.proposalThreshold,\n \u0027NounsDAO::propose: proposer votes below proposal threshold\u0027\n );\n require(\n targets.length == values.length \u0026\u0026\n targets.length == signatures.length \u0026\u0026\n targets.length == calldatas.length,\n \u0027NounsDAO::propose: proposal function information arity mismatch\u0027\n );\n require(targets.length != 0, \u0027NounsDAO::propose: must provide actions\u0027);\n require(targets.length \u003c= proposalMaxOperations, \u0027NounsDAO::propose: too many actions\u0027);\n\n temp.latestProposalId = latestProposalIds[msg.sender];\n if (temp.latestProposalId != 0) {\n ProposalState proposersLatestProposalState = state(temp.latestProposalId);\n require(\n proposersLatestProposalState != ProposalState.Active,\n \u0027NounsDAO::propose: one live proposal per proposer, found an already active proposal\u0027\n );\n require(\n proposersLatestProposalState != ProposalState.Pending,\n \u0027NounsDAO::propose: one live proposal per proposer, found an already pending proposal\u0027\n );\n }\n\n temp.startBlock = block.number + votingDelay;\n temp.endBlock = temp.startBlock + votingPeriod;\n\n proposalCount++;\n Proposal storage newProposal = _proposals[proposalCount];\n newProposal.id = proposalCount;\n newProposal.proposer = msg.sender;\n newProposal.proposalThreshold = temp.proposalThreshold;\n newProposal.eta = 0;\n newProposal.targets = targets;\n newProposal.values = values;\n newProposal.signatures = signatures;\n newProposal.calldatas = calldatas;\n newProposal.startBlock = temp.startBlock;\n newProposal.endBlock = temp.endBlock;\n newProposal.forVotes = 0;\n newProposal.againstVotes = 0;\n newProposal.abstainVotes = 0;\n newProposal.canceled = false;\n newProposal.executed = false;\n newProposal.vetoed = false;\n newProposal.totalSupply = temp.totalSupply;\n newProposal.creationBlock = block.number;\n\n latestProposalIds[newProposal.proposer] = newProposal.id;\n\n /// @notice Maintains backwards compatibility with GovernorBravo events\n emit ProposalCreated(\n newProposal.id,\n msg.sender,\n targets,\n values,\n signatures,\n calldatas,\n newProposal.startBlock,\n newProposal.endBlock,\n description\n );\n\n /// @notice Updated event with `proposalThreshold` and `minQuorumVotes`\n /// @notice `minQuorumVotes` is always zero since V2 introduces dynamic quorum with checkpoints\n emit ProposalCreatedWithRequirements(\n newProposal.id,\n msg.sender,\n targets,\n values,\n signatures,\n calldatas,\n newProposal.startBlock,\n newProposal.endBlock,\n newProposal.proposalThreshold,\n minQuorumVotes(),\n description\n );\n\n return newProposal.id;\n }\n\n /**\n * @notice Queues a proposal of state succeeded\n * @param proposalId The id of the proposal to queue\n */\n function queue(uint256 proposalId) external {\n require(\n state(proposalId) == ProposalState.Succeeded,\n \u0027NounsDAO::queue: proposal can only be queued if it is succeeded\u0027\n );\n Proposal storage proposal = _proposals[proposalId];\n uint256 eta = block.timestamp + timelock.delay();\n for (uint256 i = 0; i \u003c proposal.targets.length; i++) {\n queueOrRevertInternal(\n proposal.targets[i],\n proposal.values[i],\n proposal.signatures[i],\n proposal.calldatas[i],\n eta\n );\n }\n proposal.eta = eta;\n emit ProposalQueued(proposalId, eta);\n }\n\n function queueOrRevertInternal(\n address target,\n uint256 value,\n string memory signature,\n bytes memory data,\n uint256 eta\n ) internal {\n require(\n !timelock.queuedTransactions(keccak256(abi.encode(target, value, signature, data, eta))),\n \u0027NounsDAO::queueOrRevertInternal: identical proposal action already queued at eta\u0027\n );\n timelock.queueTransaction(target, value, signature, data, eta);\n }\n\n /**\n * @notice Executes a queued proposal if eta has passed\n * @param proposalId The id of the proposal to execute\n */\n function execute(uint256 proposalId) external {\n require(\n state(proposalId) == ProposalState.Queued,\n \u0027NounsDAO::execute: proposal can only be executed if it is queued\u0027\n );\n Proposal storage proposal = _proposals[proposalId];\n proposal.executed = true;\n for (uint256 i = 0; i \u003c proposal.targets.length; i++) {\n timelock.executeTransaction(\n proposal.targets[i],\n proposal.values[i],\n proposal.signatures[i],\n proposal.calldatas[i],\n proposal.eta\n );\n }\n emit ProposalExecuted(proposalId);\n }\n\n /**\n * @notice Cancels a proposal only if sender is the proposer, or proposer delegates dropped below proposal threshold\n * @param proposalId The id of the proposal to cancel\n */\n function cancel(uint256 proposalId) external {\n if (state(proposalId) == ProposalState.Executed) {\n revert CantCancelExecutedProposal();\n }\n\n Proposal storage proposal = _proposals[proposalId];\n require(\n msg.sender == proposal.proposer ||\n nouns.getPriorVotes(proposal.proposer, block.number - 1) \u003c= proposal.proposalThreshold,\n \u0027NounsDAO::cancel: proposer above threshold\u0027\n );\n\n proposal.canceled = true;\n for (uint256 i = 0; i \u003c proposal.targets.length; i++) {\n timelock.cancelTransaction(\n proposal.targets[i],\n proposal.values[i],\n proposal.signatures[i],\n proposal.calldatas[i],\n proposal.eta\n );\n }\n\n emit ProposalCanceled(proposalId);\n }\n\n /**\n * @notice Vetoes a proposal only if sender is the vetoer and the proposal has not been executed.\n * @param proposalId The id of the proposal to veto\n */\n function veto(uint256 proposalId) external {\n if (vetoer == address(0)) {\n revert VetoerBurned();\n }\n\n if (msg.sender != vetoer) {\n revert VetoerOnly();\n }\n\n if (state(proposalId) == ProposalState.Executed) {\n revert CantVetoExecutedProposal();\n }\n\n Proposal storage proposal = _proposals[proposalId];\n\n proposal.vetoed = true;\n for (uint256 i = 0; i \u003c proposal.targets.length; i++) {\n timelock.cancelTransaction(\n proposal.targets[i],\n proposal.values[i],\n proposal.signatures[i],\n proposal.calldatas[i],\n proposal.eta\n );\n }\n\n emit ProposalVetoed(proposalId);\n }\n\n /**\n * @notice Gets actions of a proposal\n * @param proposalId the id of the proposal\n * @return targets\n * @return values\n * @return signatures\n * @return calldatas\n */\n function getActions(uint256 proposalId)\n external\n view\n returns (\n address[] memory targets,\n uint256[] memory values,\n string[] memory signatures,\n bytes[] memory calldatas\n )\n {\n Proposal storage p = _proposals[proposalId];\n return (p.targets, p.values, p.signatures, p.calldatas);\n }\n\n /**\n * @notice Gets the receipt for a voter on a given proposal\n * @param proposalId the id of proposal\n * @param voter The address of the voter\n * @return The voting receipt\n */\n function getReceipt(uint256 proposalId, address voter) external view returns (Receipt memory) {\n return _proposals[proposalId].receipts[voter];\n }\n\n /**\n * @notice Gets the state of a proposal\n * @param proposalId The id of the proposal\n * @return Proposal state\n */\n function state(uint256 proposalId) public view returns (ProposalState) {\n require(proposalCount \u003e= proposalId, \u0027NounsDAO::state: invalid proposal id\u0027);\n Proposal storage proposal = _proposals[proposalId];\n if (proposal.vetoed) {\n return ProposalState.Vetoed;\n } else if (proposal.canceled) {\n return ProposalState.Canceled;\n } else if (block.number \u003c= proposal.startBlock) {\n return ProposalState.Pending;\n } else if (block.number \u003c= proposal.endBlock) {\n return ProposalState.Active;\n } else if (proposal.forVotes \u003c= proposal.againstVotes || proposal.forVotes \u003c quorumVotes(proposal.id)) {\n return ProposalState.Defeated;\n } else if (proposal.eta == 0) {\n return ProposalState.Succeeded;\n } else if (proposal.executed) {\n return ProposalState.Executed;\n } else if (block.timestamp \u003e= proposal.eta + timelock.GRACE_PERIOD()) {\n return ProposalState.Expired;\n } else {\n return ProposalState.Queued;\n }\n }\n\n /**\n * @notice Returns the proposal details given a proposal id.\n * The `quorumVotes` member holds the *current* quorum, given the current votes.\n * @param proposalId the proposal id to get the data for\n * @return A `ProposalCondensed` struct with the proposal data\n */\n function proposals(uint256 proposalId) external view returns (ProposalCondensed memory) {\n Proposal storage proposal = _proposals[proposalId];\n return\n ProposalCondensed({\n id: proposal.id,\n proposer: proposal.proposer,\n proposalThreshold: proposal.proposalThreshold,\n quorumVotes: quorumVotes(proposal.id),\n eta: proposal.eta,\n startBlock: proposal.startBlock,\n endBlock: proposal.endBlock,\n forVotes: proposal.forVotes,\n againstVotes: proposal.againstVotes,\n abstainVotes: proposal.abstainVotes,\n canceled: proposal.canceled,\n vetoed: proposal.vetoed,\n executed: proposal.executed,\n totalSupply: proposal.totalSupply,\n creationBlock: proposal.creationBlock\n });\n }\n\n /**\n * @notice Cast a vote for a proposal\n * @param proposalId The id of the proposal to vote on\n * @param support The support value for the vote. 0=against, 1=for, 2=abstain\n */\n function castVote(uint256 proposalId, uint8 support) external {\n emit VoteCast(msg.sender, proposalId, support, castVoteInternal(msg.sender, proposalId, support), \u0027\u0027);\n }\n\n /**\n * @notice Cast a vote for a proposal, asking the DAO to refund gas costs.\n * Users with \u003e 0 votes receive refunds. Refunds are partial when using a gas priority fee higher than the DAO\u0027s cap.\n * Refunds are partial when the DAO\u0027s balance is insufficient.\n * No refund is sent when the DAO\u0027s balance is empty. No refund is sent to users with no votes.\n * Voting takes place regardless of refund success.\n * @param proposalId The id of the proposal to vote on\n * @param support The support value for the vote. 0=against, 1=for, 2=abstain\n * @dev Reentrancy is defended against in `castVoteInternal` at the `receipt.hasVoted == false` require statement.\n */\n function castRefundableVote(uint256 proposalId, uint8 support) external {\n castRefundableVoteInternal(proposalId, support, \u0027\u0027);\n }\n\n /**\n * @notice Cast a vote for a proposal, asking the DAO to refund gas costs.\n * Users with \u003e 0 votes receive refunds. Refunds are partial when using a gas priority fee higher than the DAO\u0027s cap.\n * Refunds are partial when the DAO\u0027s balance is insufficient.\n * No refund is sent when the DAO\u0027s balance is empty. No refund is sent to users with no votes.\n * Voting takes place regardless of refund success.\n * @param proposalId The id of the proposal to vote on\n * @param support The support value for the vote. 0=against, 1=for, 2=abstain\n * @param reason The reason given for the vote by the voter\n * @dev Reentrancy is defended against in `castVoteInternal` at the `receipt.hasVoted == false` require statement.\n */\n function castRefundableVoteWithReason(\n uint256 proposalId,\n uint8 support,\n string calldata reason\n ) external {\n castRefundableVoteInternal(proposalId, support, reason);\n }\n\n /**\n * @notice Internal function that carries out refundable voting logic\n * @param proposalId The id of the proposal to vote on\n * @param support The support value for the vote. 0=against, 1=for, 2=abstain\n * @param reason The reason given for the vote by the voter\n * @dev Reentrancy is defended against in `castVoteInternal` at the `receipt.hasVoted == false` require statement.\n */\n function castRefundableVoteInternal(\n uint256 proposalId,\n uint8 support,\n string memory reason\n ) internal {\n uint256 startGas = gasleft();\n uint96 votes = castVoteInternal(msg.sender, proposalId, support);\n emit VoteCast(msg.sender, proposalId, support, votes, reason);\n if (votes \u003e 0) {\n _refundGas(startGas);\n }\n }\n\n /**\n * @notice Cast a vote for a proposal with a reason\n * @param proposalId The id of the proposal to vote on\n * @param support The support value for the vote. 0=against, 1=for, 2=abstain\n * @param reason The reason given for the vote by the voter\n */\n function castVoteWithReason(\n uint256 proposalId,\n uint8 support,\n string calldata reason\n ) external {\n emit VoteCast(msg.sender, proposalId, support, castVoteInternal(msg.sender, proposalId, support), reason);\n }\n\n /**\n * @notice Cast a vote for a proposal by signature\n * @dev External function that accepts EIP-712 signatures for voting on proposals.\n */\n function castVoteBySig(\n uint256 proposalId,\n uint8 support,\n uint8 v,\n bytes32 r,\n bytes32 s\n ) external {\n bytes32 domainSeparator = keccak256(\n abi.encode(DOMAIN_TYPEHASH, keccak256(bytes(name)), getChainIdInternal(), address(this))\n );\n bytes32 structHash = keccak256(abi.encode(BALLOT_TYPEHASH, proposalId, support));\n bytes32 digest = keccak256(abi.encodePacked(\u0027\\x19\\x01\u0027, domainSeparator, structHash));\n address signatory = ecrecover(digest, v, r, s);\n require(signatory != address(0), \u0027NounsDAO::castVoteBySig: invalid signature\u0027);\n emit VoteCast(signatory, proposalId, support, castVoteInternal(signatory, proposalId, support), \u0027\u0027);\n }\n\n /**\n * @notice Internal function that caries out voting logic\n * @param voter The voter that is casting their vote\n * @param proposalId The id of the proposal to vote on\n * @param support The support value for the vote. 0=against, 1=for, 2=abstain\n * @return The number of votes cast\n */\n function castVoteInternal(\n address voter,\n uint256 proposalId,\n uint8 support\n ) internal returns (uint96) {\n require(state(proposalId) == ProposalState.Active, \u0027NounsDAO::castVoteInternal: voting is closed\u0027);\n require(support \u003c= 2, \u0027NounsDAO::castVoteInternal: invalid vote type\u0027);\n Proposal storage proposal = _proposals[proposalId];\n Receipt storage receipt = proposal.receipts[voter];\n require(receipt.hasVoted == false, \u0027NounsDAO::castVoteInternal: voter already voted\u0027);\n\n /// @notice: Unlike GovernerBravo, votes are considered from the block the proposal was created in order to normalize quorumVotes and proposalThreshold metrics\n uint96 votes = nouns.getPriorVotes(voter, proposalCreationBlock(proposal));\n\n if (support == 0) {\n proposal.againstVotes = proposal.againstVotes + votes;\n } else if (support == 1) {\n proposal.forVotes = proposal.forVotes + votes;\n } else if (support == 2) {\n proposal.abstainVotes = proposal.abstainVotes + votes;\n }\n\n receipt.hasVoted = true;\n receipt.support = support;\n receipt.votes = votes;\n\n return votes;\n }\n\n /**\n * @notice Admin function for setting the voting delay\n * @param newVotingDelay new voting delay, in blocks\n */\n function _setVotingDelay(uint256 newVotingDelay) external {\n if (msg.sender != admin) {\n revert AdminOnly();\n }\n require(\n newVotingDelay \u003e= MIN_VOTING_DELAY \u0026\u0026 newVotingDelay \u003c= MAX_VOTING_DELAY,\n \u0027NounsDAO::_setVotingDelay: invalid voting delay\u0027\n );\n uint256 oldVotingDelay = votingDelay;\n votingDelay = newVotingDelay;\n\n emit VotingDelaySet(oldVotingDelay, votingDelay);\n }\n\n /**\n * @notice Admin function for setting the voting period\n * @param newVotingPeriod new voting period, in blocks\n */\n function _setVotingPeriod(uint256 newVotingPeriod) external {\n if (msg.sender != admin) {\n revert AdminOnly();\n }\n require(\n newVotingPeriod \u003e= MIN_VOTING_PERIOD \u0026\u0026 newVotingPeriod \u003c= MAX_VOTING_PERIOD,\n \u0027NounsDAO::_setVotingPeriod: invalid voting period\u0027\n );\n uint256 oldVotingPeriod = votingPeriod;\n votingPeriod = newVotingPeriod;\n\n emit VotingPeriodSet(oldVotingPeriod, votingPeriod);\n }\n\n /**\n * @notice Admin function for setting the proposal threshold basis points\n * @dev newProposalThresholdBPS must be greater than the hardcoded min\n * @param newProposalThresholdBPS new proposal threshold\n */\n function _setProposalThresholdBPS(uint256 newProposalThresholdBPS) external {\n if (msg.sender != admin) {\n revert AdminOnly();\n }\n require(\n newProposalThresholdBPS \u003e= MIN_PROPOSAL_THRESHOLD_BPS \u0026\u0026\n newProposalThresholdBPS \u003c= MAX_PROPOSAL_THRESHOLD_BPS,\n \u0027NounsDAO::_setProposalThreshold: invalid proposal threshold bps\u0027\n );\n uint256 oldProposalThresholdBPS = proposalThresholdBPS;\n proposalThresholdBPS = newProposalThresholdBPS;\n\n emit ProposalThresholdBPSSet(oldProposalThresholdBPS, proposalThresholdBPS);\n }\n\n /**\n * @notice Admin function for setting the minimum quorum votes bps\n * @param newMinQuorumVotesBPS minimum quorum votes bps\n * Must be between `MIN_QUORUM_VOTES_BPS_LOWER_BOUND` and `MIN_QUORUM_VOTES_BPS_UPPER_BOUND`\n * Must be lower than or equal to maxQuorumVotesBPS\n */\n function _setMinQuorumVotesBPS(uint16 newMinQuorumVotesBPS) external {\n if (msg.sender != admin) {\n revert AdminOnly();\n }\n DynamicQuorumParams memory params = getDynamicQuorumParamsAt(block.number);\n\n require(\n newMinQuorumVotesBPS \u003e= MIN_QUORUM_VOTES_BPS_LOWER_BOUND \u0026\u0026\n newMinQuorumVotesBPS \u003c= MIN_QUORUM_VOTES_BPS_UPPER_BOUND,\n \u0027NounsDAO::_setMinQuorumVotesBPS: invalid min quorum votes bps\u0027\n );\n require(\n newMinQuorumVotesBPS \u003c= params.maxQuorumVotesBPS,\n \u0027NounsDAO::_setMinQuorumVotesBPS: min quorum votes bps greater than max\u0027\n );\n\n uint16 oldMinQuorumVotesBPS = params.minQuorumVotesBPS;\n params.minQuorumVotesBPS = newMinQuorumVotesBPS;\n\n _writeQuorumParamsCheckpoint(params);\n\n emit MinQuorumVotesBPSSet(oldMinQuorumVotesBPS, newMinQuorumVotesBPS);\n }\n\n /**\n * @notice Admin function for setting the maximum quorum votes bps\n * @param newMaxQuorumVotesBPS maximum quorum votes bps\n * Must be lower than `MAX_QUORUM_VOTES_BPS_UPPER_BOUND`\n * Must be higher than or equal to minQuorumVotesBPS\n */\n function _setMaxQuorumVotesBPS(uint16 newMaxQuorumVotesBPS) external {\n if (msg.sender != admin) {\n revert AdminOnly();\n }\n DynamicQuorumParams memory params = getDynamicQuorumParamsAt(block.number);\n\n require(\n newMaxQuorumVotesBPS \u003c= MAX_QUORUM_VOTES_BPS_UPPER_BOUND,\n \u0027NounsDAO::_setMaxQuorumVotesBPS: invalid max quorum votes bps\u0027\n );\n require(\n params.minQuorumVotesBPS \u003c= newMaxQuorumVotesBPS,\n \u0027NounsDAO::_setMaxQuorumVotesBPS: min quorum votes bps greater than max\u0027\n );\n\n uint16 oldMaxQuorumVotesBPS = params.maxQuorumVotesBPS;\n params.maxQuorumVotesBPS = newMaxQuorumVotesBPS;\n\n _writeQuorumParamsCheckpoint(params);\n\n emit MaxQuorumVotesBPSSet(oldMaxQuorumVotesBPS, newMaxQuorumVotesBPS);\n }\n\n /**\n * @notice Admin function for setting the dynamic quorum coefficient\n * @param newQuorumCoefficient the new coefficient, as a fixed point integer with 6 decimals\n */\n function _setQuorumCoefficient(uint32 newQuorumCoefficient) external {\n if (msg.sender != admin) {\n revert AdminOnly();\n }\n DynamicQuorumParams memory params = getDynamicQuorumParamsAt(block.number);\n\n uint32 oldQuorumCoefficient = params.quorumCoefficient;\n params.quorumCoefficient = newQuorumCoefficient;\n\n _writeQuorumParamsCheckpoint(params);\n\n emit QuorumCoefficientSet(oldQuorumCoefficient, newQuorumCoefficient);\n }\n\n /**\n * @notice Admin function for setting all the dynamic quorum parameters\n * @param newMinQuorumVotesBPS minimum quorum votes bps\n * Must be between `MIN_QUORUM_VOTES_BPS_LOWER_BOUND` and `MIN_QUORUM_VOTES_BPS_UPPER_BOUND`\n * Must be lower than or equal to maxQuorumVotesBPS\n * @param newMaxQuorumVotesBPS maximum quorum votes bps\n * Must be lower than `MAX_QUORUM_VOTES_BPS_UPPER_BOUND`\n * Must be higher than or equal to minQuorumVotesBPS\n * @param newQuorumCoefficient the new coefficient, as a fixed point integer with 6 decimals\n */\n function _setDynamicQuorumParams(\n uint16 newMinQuorumVotesBPS,\n uint16 newMaxQuorumVotesBPS,\n uint32 newQuorumCoefficient\n ) public {\n if (msg.sender != admin) {\n revert AdminOnly();\n }\n if (\n newMinQuorumVotesBPS \u003c MIN_QUORUM_VOTES_BPS_LOWER_BOUND ||\n newMinQuorumVotesBPS \u003e MIN_QUORUM_VOTES_BPS_UPPER_BOUND\n ) {\n revert InvalidMinQuorumVotesBPS();\n }\n if (newMaxQuorumVotesBPS \u003e MAX_QUORUM_VOTES_BPS_UPPER_BOUND) {\n revert InvalidMaxQuorumVotesBPS();\n }\n if (newMinQuorumVotesBPS \u003e newMaxQuorumVotesBPS) {\n revert MinQuorumBPSGreaterThanMaxQuorumBPS();\n }\n\n DynamicQuorumParams memory oldParams = getDynamicQuorumParamsAt(block.number);\n\n DynamicQuorumParams memory params = DynamicQuorumParams({\n minQuorumVotesBPS: newMinQuorumVotesBPS,\n maxQuorumVotesBPS: newMaxQuorumVotesBPS,\n quorumCoefficient: newQuorumCoefficient\n });\n _writeQuorumParamsCheckpoint(params);\n\n emit MinQuorumVotesBPSSet(oldParams.minQuorumVotesBPS, params.minQuorumVotesBPS);\n emit MaxQuorumVotesBPSSet(oldParams.maxQuorumVotesBPS, params.maxQuorumVotesBPS);\n emit QuorumCoefficientSet(oldParams.quorumCoefficient, params.quorumCoefficient);\n }\n\n function _withdraw() external returns (uint256, bool) {\n if (msg.sender != admin) {\n revert AdminOnly();\n }\n\n uint256 amount = address(this).balance;\n (bool sent, ) = msg.sender.call{ value: amount }(\u0027\u0027);\n\n emit Withdraw(amount, sent);\n\n return (amount, sent);\n }\n\n /**\n * @notice Begins transfer of admin rights. The newPendingAdmin must call `_acceptAdmin` to finalize the transfer.\n * @dev Admin function to begin change of admin. The newPendingAdmin must call `_acceptAdmin` to finalize the transfer.\n * @param newPendingAdmin New pending admin.\n */\n function _setPendingAdmin(address newPendingAdmin) external {\n // Check caller = admin\n require(msg.sender == admin, \u0027NounsDAO::_setPendingAdmin: admin only\u0027);\n\n // Save current value, if any, for inclusion in log\n address oldPendingAdmin = pendingAdmin;\n\n // Store pendingAdmin with value newPendingAdmin\n pendingAdmin = newPendingAdmin;\n\n // Emit NewPendingAdmin(oldPendingAdmin, newPendingAdmin)\n emit NewPendingAdmin(oldPendingAdmin, newPendingAdmin);\n }\n\n /**\n * @notice Accepts transfer of admin rights. msg.sender must be pendingAdmin\n * @dev Admin function for pending admin to accept role and update admin\n */\n function _acceptAdmin() external {\n // Check caller is pendingAdmin and pendingAdmin ≠ address(0)\n require(msg.sender == pendingAdmin \u0026\u0026 msg.sender != address(0), \u0027NounsDAO::_acceptAdmin: pending admin only\u0027);\n\n // Save current values for inclusion in log\n address oldAdmin = admin;\n address oldPendingAdmin = pendingAdmin;\n\n // Store admin with value pendingAdmin\n admin = pendingAdmin;\n\n // Clear the pending value\n pendingAdmin = address(0);\n\n emit NewAdmin(oldAdmin, admin);\n emit NewPendingAdmin(oldPendingAdmin, pendingAdmin);\n }\n\n /**\n * @notice Begins transition of vetoer. The newPendingVetoer must call _acceptVetoer to finalize the transfer.\n * @param newPendingVetoer New Pending Vetoer\n */\n function _setPendingVetoer(address newPendingVetoer) public {\n if (msg.sender != vetoer) {\n revert VetoerOnly();\n }\n\n emit NewPendingVetoer(pendingVetoer, newPendingVetoer);\n\n pendingVetoer = newPendingVetoer;\n }\n\n function _acceptVetoer() external {\n if (msg.sender != pendingVetoer) {\n revert PendingVetoerOnly();\n }\n\n // Update vetoer\n emit NewVetoer(vetoer, pendingVetoer);\n vetoer = pendingVetoer;\n\n // Clear the pending value\n emit NewPendingVetoer(pendingVetoer, address(0));\n pendingVetoer = address(0);\n }\n\n /**\n * @notice Burns veto priviledges\n * @dev Vetoer function destroying veto power forever\n */\n function _burnVetoPower() public {\n // Check caller is vetoer\n require(msg.sender == vetoer, \u0027NounsDAO::_burnVetoPower: vetoer only\u0027);\n\n // Update vetoer to 0x0\n emit NewVetoer(vetoer, address(0));\n vetoer = address(0);\n\n // Clear the pending value\n emit NewPendingVetoer(pendingVetoer, address(0));\n pendingVetoer = address(0);\n }\n\n /**\n * @notice Current proposal threshold using Noun Total Supply\n * Differs from `GovernerBravo` which uses fixed amount\n */\n function proposalThreshold() public view returns (uint256) {\n return bps2Uint(proposalThresholdBPS, nouns.totalSupply());\n }\n\n function proposalCreationBlock(Proposal storage proposal) internal view returns (uint256) {\n if (proposal.creationBlock == 0) {\n return proposal.startBlock - votingDelay;\n }\n return proposal.creationBlock;\n }\n\n /**\n * @notice Quorum votes required for a specific proposal to succeed\n * Differs from `GovernerBravo` which uses fixed amount\n */\n function quorumVotes(uint256 proposalId) public view returns (uint256) {\n Proposal storage proposal = _proposals[proposalId];\n if (proposal.totalSupply == 0) {\n return proposal.quorumVotes;\n }\n\n return\n dynamicQuorumVotes(\n proposal.againstVotes,\n proposal.totalSupply,\n getDynamicQuorumParamsAt(proposal.creationBlock)\n );\n }\n\n /**\n * @notice Calculates the required quorum of for-votes based on the amount of against-votes\n * The more against-votes there are for a proposal, the higher the required quorum is.\n * The quorum BPS is between `params.minQuorumVotesBPS` and params.maxQuorumVotesBPS.\n * The additional quorum is calculated as:\n * quorumCoefficient * againstVotesBPS\n * @dev Note the coefficient is a fixed point integer with 6 decimals\n * @param againstVotes Number of against-votes in the proposal\n * @param totalSupply The total supply of Nouns at the time of proposal creation\n * @param params Configurable parameters for calculating the quorum based on againstVotes. See `DynamicQuorumParams` definition for additional details.\n * @return quorumVotes The required quorum\n */\n function dynamicQuorumVotes(\n uint256 againstVotes,\n uint256 totalSupply,\n DynamicQuorumParams memory params\n ) public pure returns (uint256) {\n uint256 againstVotesBPS = (10000 * againstVotes) / totalSupply;\n uint256 quorumAdjustmentBPS = (params.quorumCoefficient * againstVotesBPS) / 1e6;\n uint256 adjustedQuorumBPS = params.minQuorumVotesBPS + quorumAdjustmentBPS;\n uint256 quorumBPS = min(params.maxQuorumVotesBPS, adjustedQuorumBPS);\n return bps2Uint(quorumBPS, totalSupply);\n }\n\n /**\n * @notice returns the dynamic quorum parameters values at a certain block number\n * @dev The checkpoints array must not be empty, and the block number must be higher than or equal to\n * the block of the first checkpoint\n * @param blockNumber_ the block number to get the params at\n * @return The dynamic quorum parameters that were set at the given block number\n */\n function getDynamicQuorumParamsAt(uint256 blockNumber_) public view returns (DynamicQuorumParams memory) {\n uint32 blockNumber = safe32(blockNumber_, \u0027NounsDAO::getDynamicQuorumParamsAt: block number exceeds 32 bits\u0027);\n uint256 len = quorumParamsCheckpoints.length;\n\n if (len == 0) {\n return\n DynamicQuorumParams({\n minQuorumVotesBPS: safe16(quorumVotesBPS),\n maxQuorumVotesBPS: safe16(quorumVotesBPS),\n quorumCoefficient: 0\n });\n }\n\n if (quorumParamsCheckpoints[len - 1].fromBlock \u003c= blockNumber) {\n return quorumParamsCheckpoints[len - 1].params;\n }\n\n if (quorumParamsCheckpoints[0].fromBlock \u003e blockNumber) {\n return\n DynamicQuorumParams({\n minQuorumVotesBPS: safe16(quorumVotesBPS),\n maxQuorumVotesBPS: safe16(quorumVotesBPS),\n quorumCoefficient: 0\n });\n }\n\n uint256 lower = 0;\n uint256 upper = len - 1;\n while (upper \u003e lower) {\n uint256 center = upper - (upper - lower) / 2;\n DynamicQuorumParamsCheckpoint memory cp = quorumParamsCheckpoints[center];\n if (cp.fromBlock == blockNumber) {\n return cp.params;\n } else if (cp.fromBlock \u003c blockNumber) {\n lower = center;\n } else {\n upper = center - 1;\n }\n }\n return quorumParamsCheckpoints[lower].params;\n }\n\n function _writeQuorumParamsCheckpoint(DynamicQuorumParams memory params) internal {\n uint32 blockNumber = safe32(block.number, \u0027block number exceeds 32 bits\u0027);\n uint256 pos = quorumParamsCheckpoints.length;\n if (pos \u003e 0 \u0026\u0026 quorumParamsCheckpoints[pos - 1].fromBlock == blockNumber) {\n quorumParamsCheckpoints[pos - 1].params = params;\n } else {\n quorumParamsCheckpoints.push(DynamicQuorumParamsCheckpoint({ fromBlock: blockNumber, params: params }));\n }\n }\n\n function _refundGas(uint256 startGas) internal {\n unchecked {\n uint256 balance = address(this).balance;\n if (balance == 0) {\n return;\n }\n uint256 basefee = min(block.basefee, MAX_REFUND_BASE_FEE);\n uint256 gasPrice = min(tx.gasprice, basefee + MAX_REFUND_PRIORITY_FEE);\n uint256 gasUsed = min(startGas - gasleft() + REFUND_BASE_GAS, MAX_REFUND_GAS_USED);\n uint256 refundAmount = min(gasPrice * gasUsed, balance);\n (bool refundSent, ) = tx.origin.call{ value: refundAmount }(\u0027\u0027);\n emit RefundableVote(tx.origin, refundAmount, refundSent);\n }\n }\n\n function min(uint256 a, uint256 b) internal pure returns (uint256) {\n return a \u003c b ? a : b;\n }\n\n /**\n * @notice Current min quorum votes using Noun total supply\n */\n function minQuorumVotes() public view returns (uint256) {\n return bps2Uint(getDynamicQuorumParamsAt(block.number).minQuorumVotesBPS, nouns.totalSupply());\n }\n\n /**\n * @notice Current max quorum votes using Noun total supply\n */\n function maxQuorumVotes() public view returns (uint256) {\n return bps2Uint(getDynamicQuorumParamsAt(block.number).maxQuorumVotesBPS, nouns.totalSupply());\n }\n\n function bps2Uint(uint256 bps, uint256 number) internal pure returns (uint256) {\n return (number * bps) / 10000;\n }\n\n function getChainIdInternal() internal view returns (uint256) {\n uint256 chainId;\n assembly {\n chainId := chainid()\n }\n return chainId;\n }\n\n function safe32(uint256 n, string memory errorMessage) internal pure returns (uint32) {\n require(n \u003c= type(uint32).max, errorMessage);\n return uint32(n);\n }\n\n function safe16(uint256 n) internal pure returns (uint16) {\n if (n \u003e type(uint16).max) {\n revert UnsafeUint16Cast();\n }\n return uint16(n);\n }\n\n receive() external payable {}\n}\n"}}
File 3 of 3: NounsToken
// SPDX-License-Identifier: GPL-3.0 /// @title The Nouns ERC-721 token /********************************* * ░░░░░░░░░░░░░░░░░░░░░░░░░░░░░ * * ░░░░░░░░░░░░░░░░░░░░░░░░░░░░░ * * ░░░░░░█████████░░█████████░░░ * * ░░░░░░██░░░████░░██░░░████░░░ * * ░░██████░░░████████░░░████░░░ * * ░░██░░██░░░████░░██░░░████░░░ * * ░░██░░██░░░████░░██░░░████░░░ * * ░░░░░░█████████░░█████████░░░ * * ░░░░░░░░░░░░░░░░░░░░░░░░░░░░░ * * ░░░░░░░░░░░░░░░░░░░░░░░░░░░░░ * *********************************/ pragma solidity ^0.8.6; import { Ownable } from '@openzeppelin/contracts/access/Ownable.sol'; import { ERC721Checkpointable } from './base/ERC721Checkpointable.sol'; import { INounsDescriptor } from './interfaces/INounsDescriptor.sol'; import { INounsSeeder } from './interfaces/INounsSeeder.sol'; import { INounsToken } from './interfaces/INounsToken.sol'; import { ERC721 } from './base/ERC721.sol'; import { IERC721 } from '@openzeppelin/contracts/token/ERC721/IERC721.sol'; import { IProxyRegistry } from './external/opensea/IProxyRegistry.sol'; contract NounsToken is INounsToken, Ownable, ERC721Checkpointable { // The lilnounders DAO address (creators org) address public lilnoundersDAO; // The nouns DAO address address public nounsDAO; // An address who has permissions to mint Nouns address public minter; // The Nouns token URI descriptor INounsDescriptor public descriptor; // The Nouns token seeder INounsSeeder public seeder; // Whether the minter can be updated bool public isMinterLocked; // Whether the descriptor can be updated bool public isDescriptorLocked; // Whether the seeder can be updated bool public isSeederLocked; // The noun seeds mapping(uint256 => INounsSeeder.Seed) public seeds; // The internal noun ID tracker uint256 private _currentNounId; // IPFS content hash of contract-level metadata string private _contractURIHash = 'QmNPz2kfXLJwYo1AFQnmu6EjeXraz2iExvCSbENqwr5aFy'; // OpenSea's Proxy Registry IProxyRegistry public immutable proxyRegistry; /** * @notice Require that the minter has not been locked. */ modifier whenMinterNotLocked() { require(!isMinterLocked, 'Minter is locked'); _; } /** * @notice Require that the descriptor has not been locked. */ modifier whenDescriptorNotLocked() { require(!isDescriptorLocked, 'Descriptor is locked'); _; } /** * @notice Require that the seeder has not been locked. */ modifier whenSeederNotLocked() { require(!isSeederLocked, 'Seeder is locked'); _; } /** * @notice Require that the sender is the nouns DAO. */ modifier onlyNounsDAO() { require(msg.sender == nounsDAO, 'Sender is not the nouns DAO'); _; } /** * @notice Require that the sender is the lil nounders DAO. */ modifier onlyLilNoundersDAO() { require(msg.sender == lilnoundersDAO, 'Sender is not the lil nounders DAO'); _; } /** * @notice Require that the sender is the minter. */ modifier onlyMinter() { require(msg.sender == minter, 'Sender is not the minter'); _; } constructor( address _lilnoundersDAO, address _nounsDAO, address _minter, INounsDescriptor _descriptor, INounsSeeder _seeder, IProxyRegistry _proxyRegistry ) ERC721("LilNoun", "LILNOUN") { lilnoundersDAO = _lilnoundersDAO; nounsDAO = _nounsDAO; minter = _minter; descriptor = _descriptor; seeder = _seeder; proxyRegistry = _proxyRegistry; } /** * @notice The IPFS URI of contract-level metadata. */ function contractURI() public view returns (string memory) { return string(abi.encodePacked('ipfs://', _contractURIHash)); } /** * @notice Set the _contractURIHash. * @dev Only callable by the owner. */ function setContractURIHash(string memory newContractURIHash) external onlyOwner { _contractURIHash = newContractURIHash; } /** * @notice Override isApprovedForAll to whitelist user's OpenSea proxy accounts to enable gas-less listings. */ function isApprovedForAll(address owner, address operator) public view override(IERC721, ERC721) returns (bool) { // Whitelist OpenSea proxy contract for easy trading. if (proxyRegistry.proxies(owner) == operator) { return true; } return super.isApprovedForAll(owner, operator); } /** * @notice Mint a Noun to the minter, along with a possible lilnounders and NounsDAO reward * Nouns. Lil Nounders reward Nouns are minted every 10 Nouns, * starting at 0, until 183 lil nounder Nouns have been minted (5 years w/ 24 hour auctions). * starting at 0, until 17520 lil nounder Nouns have been minted (5 years w/ 24 hour auctions). * starting at 0, until 8760 lil nounder Nouns have been minted (5 years w/ 24 hour auctions). * @dev Call _mintTo with the to address(es). */ function mint() public override onlyMinter returns (uint256) { if (_currentNounId <= 175300 && _currentNounId % 10 == 0) { _mintTo(lilnoundersDAO, _currentNounId++); } if (_currentNounId <= 175301 && _currentNounId % 10 == 1) { _mintTo(nounsDAO, _currentNounId++); } return _mintTo(minter, _currentNounId++); } /** * @notice Burn a noun. */ function burn(uint256 nounId) public override onlyMinter { _burn(nounId); emit NounBurned(nounId); } /** * @notice A distinct Uniform Resource Identifier (URI) for a given asset. * @dev See {IERC721Metadata-tokenURI}. */ function tokenURI(uint256 tokenId) public view override returns (string memory) { require(_exists(tokenId), 'NounsToken: URI query for nonexistent token'); return descriptor.tokenURI(tokenId, seeds[tokenId]); } /** * @notice Similar to `tokenURI`, but always serves a base64 encoded data URI * with the JSON contents directly inlined. */ function dataURI(uint256 tokenId) public view override returns (string memory) { require(_exists(tokenId), 'NounsToken: URI query for nonexistent token'); return descriptor.dataURI(tokenId, seeds[tokenId]); } /** * @notice Set the nouns DAO. * @dev Only callable by the nouns DAO when not locked. */ function setNounsDAO(address _nounsDAO) external override onlyNounsDAO { nounsDAO = _nounsDAO; emit NounsDAOUpdated(_nounsDAO); } /** * @notice Set the lil nounders DAO. * @dev Only callable by the lilnounders DAO when not locked. */ function setLilNoundersDAO(address _lilnoundersDAO) external override onlyLilNoundersDAO { lilnoundersDAO = _lilnoundersDAO; emit LilNoundersDAOUpdated(_lilnoundersDAO); } /** * @notice Set the token minter. * @dev Only callable by the owner when not locked. */ function setMinter(address _minter) external override onlyOwner whenMinterNotLocked { minter = _minter; emit MinterUpdated(_minter); } /** * @notice Lock the minter. * @dev This cannot be reversed and is only callable by the owner when not locked. */ function lockMinter() external override onlyOwner whenMinterNotLocked { isMinterLocked = true; emit MinterLocked(); } /** * @notice Set the token URI descriptor. * @dev Only callable by the owner when not locked. */ function setDescriptor(INounsDescriptor _descriptor) external override onlyOwner whenDescriptorNotLocked { descriptor = _descriptor; emit DescriptorUpdated(_descriptor); } /** * @notice Lock the descriptor. * @dev This cannot be reversed and is only callable by the owner when not locked. */ function lockDescriptor() external override onlyOwner whenDescriptorNotLocked { isDescriptorLocked = true; emit DescriptorLocked(); } /** * @notice Set the token seeder. * @dev Only callable by the owner when not locked. */ function setSeeder(INounsSeeder _seeder) external override onlyOwner whenSeederNotLocked { seeder = _seeder; emit SeederUpdated(_seeder); } /** * @notice Lock the seeder. * @dev This cannot be reversed and is only callable by the owner when not locked. */ function lockSeeder() external override onlyOwner whenSeederNotLocked { isSeederLocked = true; emit SeederLocked(); } /** * @notice Mint a Noun with `nounId` to the provided `to` address. */ function _mintTo(address to, uint256 nounId) internal returns (uint256) { INounsSeeder.Seed memory seed = seeds[nounId] = seeder.generateSeed(nounId, descriptor); _mint(owner(), to, nounId); emit NounCreated(nounId, seed); return nounId; } } // SPDX-License-Identifier: MIT // OpenZeppelin Contracts v4.4.0 (access/Ownable.sol) pragma solidity ^0.8.0; import "../utils/Context.sol"; /** * @dev Contract module which provides a basic access control mechanism, where * there is an account (an owner) that can be granted exclusive access to * specific functions. * * By default, the owner account will be the one that deploys the contract. This * can later be changed with {transferOwnership}. * * This module is used through inheritance. It will make available the modifier * `onlyOwner`, which can be applied to your functions to restrict their use to * the owner. */ abstract contract Ownable is Context { address private _owner; event OwnershipTransferred(address indexed previousOwner, address indexed newOwner); /** * @dev Initializes the contract setting the deployer as the initial owner. */ constructor() { _transferOwnership(_msgSender()); } /** * @dev Returns the address of the current owner. */ function owner() public view virtual returns (address) { return _owner; } /** * @dev Throws if called by any account other than the owner. */ modifier onlyOwner() { require(owner() == _msgSender(), "Ownable: caller is not the owner"); _; } /** * @dev Leaves the contract without owner. It will not be possible to call * `onlyOwner` functions anymore. Can only be called by the current owner. * * NOTE: Renouncing ownership will leave the contract without an owner, * thereby removing any functionality that is only available to the owner. */ function renounceOwnership() public virtual onlyOwner { _transferOwnership(address(0)); } /** * @dev Transfers ownership of the contract to a new account (`newOwner`). * Can only be called by the current owner. */ function transferOwnership(address newOwner) public virtual onlyOwner { require(newOwner != address(0), "Ownable: new owner is the zero address"); _transferOwnership(newOwner); } /** * @dev Transfers ownership of the contract to a new account (`newOwner`). * Internal function without access restriction. */ function _transferOwnership(address newOwner) internal virtual { address oldOwner = _owner; _owner = newOwner; emit OwnershipTransferred(oldOwner, newOwner); } } // SPDX-License-Identifier: BSD-3-Clause /// @title Vote checkpointing for an ERC-721 token /********************************* * ░░░░░░░░░░░░░░░░░░░░░░░░░░░░░ * * ░░░░░░░░░░░░░░░░░░░░░░░░░░░░░ * * ░░░░░░█████████░░█████████░░░ * * ░░░░░░██░░░████░░██░░░████░░░ * * ░░██████░░░████████░░░████░░░ * * ░░██░░██░░░████░░██░░░████░░░ * * ░░██░░██░░░████░░██░░░████░░░ * * ░░░░░░█████████░░█████████░░░ * * ░░░░░░░░░░░░░░░░░░░░░░░░░░░░░ * * ░░░░░░░░░░░░░░░░░░░░░░░░░░░░░ * *********************************/ // LICENSE // ERC721Checkpointable.sol uses and modifies part of Compound Lab's Comp.sol: // https://github.com/compound-finance/compound-protocol/blob/ae4388e780a8d596d97619d9704a931a2752c2bc/contracts/Governance/Comp.sol // // Comp.sol source code Copyright 2020 Compound Labs, Inc. licensed under the BSD-3-Clause license. // With modifications by Nounders DAO. // // Additional conditions of BSD-3-Clause can be found here: https://opensource.org/licenses/BSD-3-Clause // // MODIFICATIONS // Checkpointing logic from Comp.sol has been used with the following modifications: // - `delegates` is renamed to `_delegates` and is set to private // - `delegates` is a public function that uses the `_delegates` mapping look-up, but unlike // Comp.sol, returns the delegator's own address if there is no delegate. // This avoids the delegator needing to "delegate to self" with an additional transaction // - `_transferTokens()` is renamed `_beforeTokenTransfer()` and adapted to hook into OpenZeppelin's ERC721 hooks. pragma solidity ^0.8.6; import './ERC721Enumerable.sol'; abstract contract ERC721Checkpointable is ERC721Enumerable { /// @notice Defines decimals as per ERC-20 convention to make integrations with 3rd party governance platforms easier uint8 public constant decimals = 0; /// @notice A record of each accounts delegate mapping(address => address) private _delegates; /// @notice A checkpoint for marking number of votes from a given block struct Checkpoint { uint32 fromBlock; uint96 votes; } /// @notice A record of votes checkpoints for each account, by index mapping(address => mapping(uint32 => Checkpoint)) public checkpoints; /// @notice The number of checkpoints for each account mapping(address => uint32) public numCheckpoints; /// @notice The EIP-712 typehash for the contract's domain bytes32 public constant DOMAIN_TYPEHASH = keccak256('EIP712Domain(string name,uint256 chainId,address verifyingContract)'); /// @notice The EIP-712 typehash for the delegation struct used by the contract bytes32 public constant DELEGATION_TYPEHASH = keccak256('Delegation(address delegatee,uint256 nonce,uint256 expiry)'); /// @notice A record of states for signing / validating signatures mapping(address => uint256) public nonces; /// @notice An event thats emitted when an account changes its delegate event DelegateChanged(address indexed delegator, address indexed fromDelegate, address indexed toDelegate); /// @notice An event thats emitted when a delegate account's vote balance changes event DelegateVotesChanged(address indexed delegate, uint256 previousBalance, uint256 newBalance); /** * @notice The votes a delegator can delegate, which is the current balance of the delegator. * @dev Used when calling `_delegate()` */ function votesToDelegate(address delegator) public view returns (uint96) { return safe96(balanceOf(delegator), 'ERC721Checkpointable::votesToDelegate: amount exceeds 96 bits'); } /** * @notice Overrides the standard `Comp.sol` delegates mapping to return * the delegator's own address if they haven't delegated. * This avoids having to delegate to oneself. */ function delegates(address delegator) public view returns (address) { address current = _delegates[delegator]; return current == address(0) ? delegator : current; } /** * @notice Adapted from `_transferTokens()` in `Comp.sol` to update delegate votes. * @dev hooks into OpenZeppelin's `ERC721._transfer` */ function _beforeTokenTransfer( address from, address to, uint256 tokenId ) internal override { super._beforeTokenTransfer(from, to, tokenId); /// @notice Differs from `_transferTokens()` to use `delegates` override method to simulate auto-delegation _moveDelegates(delegates(from), delegates(to), 1); } /** * @notice Delegate votes from `msg.sender` to `delegatee` * @param delegatee The address to delegate votes to */ function delegate(address delegatee) public { if (delegatee == address(0)) delegatee = msg.sender; return _delegate(msg.sender, delegatee); } /** * @notice Delegates votes from signatory to `delegatee` * @param delegatee The address to delegate votes to * @param nonce The contract state required to match the signature * @param expiry The time at which to expire the signature * @param v The recovery byte of the signature * @param r Half of the ECDSA signature pair * @param s Half of the ECDSA signature pair */ function delegateBySig( address delegatee, uint256 nonce, uint256 expiry, uint8 v, bytes32 r, bytes32 s ) public { bytes32 domainSeparator = keccak256( abi.encode(DOMAIN_TYPEHASH, keccak256(bytes(name())), getChainId(), address(this)) ); bytes32 structHash = keccak256(abi.encode(DELEGATION_TYPEHASH, delegatee, nonce, expiry)); bytes32 digest = keccak256(abi.encodePacked('\\x19\\x01', domainSeparator, structHash)); address signatory = ecrecover(digest, v, r, s); require(signatory != address(0), 'ERC721Checkpointable::delegateBySig: invalid signature'); require(nonce == nonces[signatory]++, 'ERC721Checkpointable::delegateBySig: invalid nonce'); require(block.timestamp <= expiry, 'ERC721Checkpointable::delegateBySig: signature expired'); return _delegate(signatory, delegatee); } /** * @notice Gets the current votes balance for `account` * @param account The address to get votes balance * @return The number of current votes for `account` */ function getCurrentVotes(address account) external view returns (uint96) { uint32 nCheckpoints = numCheckpoints[account]; return nCheckpoints > 0 ? checkpoints[account][nCheckpoints - 1].votes : 0; } /** * @notice Determine the prior number of votes for an account as of a block number * @dev Block number must be a finalized block or else this function will revert to prevent misinformation. * @param account The address of the account to check * @param blockNumber The block number to get the vote balance at * @return The number of votes the account had as of the given block */ function getPriorVotes(address account, uint256 blockNumber) public view returns (uint96) { require(blockNumber < block.number, 'ERC721Checkpointable::getPriorVotes: not yet determined'); uint32 nCheckpoints = numCheckpoints[account]; if (nCheckpoints == 0) { return 0; } // First check most recent balance if (checkpoints[account][nCheckpoints - 1].fromBlock <= blockNumber) { return checkpoints[account][nCheckpoints - 1].votes; } // Next check implicit zero balance if (checkpoints[account][0].fromBlock > blockNumber) { return 0; } uint32 lower = 0; uint32 upper = nCheckpoints - 1; while (upper > lower) { uint32 center = upper - (upper - lower) / 2; // ceil, avoiding overflow Checkpoint memory cp = checkpoints[account][center]; if (cp.fromBlock == blockNumber) { return cp.votes; } else if (cp.fromBlock < blockNumber) { lower = center; } else { upper = center - 1; } } return checkpoints[account][lower].votes; } function _delegate(address delegator, address delegatee) internal { /// @notice differs from `_delegate()` in `Comp.sol` to use `delegates` override method to simulate auto-delegation address currentDelegate = delegates(delegator); _delegates[delegator] = delegatee; emit DelegateChanged(delegator, currentDelegate, delegatee); uint96 amount = votesToDelegate(delegator); _moveDelegates(currentDelegate, delegatee, amount); } function _moveDelegates( address srcRep, address dstRep, uint96 amount ) internal { if (srcRep != dstRep && amount > 0) { if (srcRep != address(0)) { uint32 srcRepNum = numCheckpoints[srcRep]; uint96 srcRepOld = srcRepNum > 0 ? checkpoints[srcRep][srcRepNum - 1].votes : 0; uint96 srcRepNew = sub96(srcRepOld, amount, 'ERC721Checkpointable::_moveDelegates: amount underflows'); _writeCheckpoint(srcRep, srcRepNum, srcRepOld, srcRepNew); } if (dstRep != address(0)) { uint32 dstRepNum = numCheckpoints[dstRep]; uint96 dstRepOld = dstRepNum > 0 ? checkpoints[dstRep][dstRepNum - 1].votes : 0; uint96 dstRepNew = add96(dstRepOld, amount, 'ERC721Checkpointable::_moveDelegates: amount overflows'); _writeCheckpoint(dstRep, dstRepNum, dstRepOld, dstRepNew); } } } function _writeCheckpoint( address delegatee, uint32 nCheckpoints, uint96 oldVotes, uint96 newVotes ) internal { uint32 blockNumber = safe32( block.number, 'ERC721Checkpointable::_writeCheckpoint: block number exceeds 32 bits' ); if (nCheckpoints > 0 && checkpoints[delegatee][nCheckpoints - 1].fromBlock == blockNumber) { checkpoints[delegatee][nCheckpoints - 1].votes = newVotes; } else { checkpoints[delegatee][nCheckpoints] = Checkpoint(blockNumber, newVotes); numCheckpoints[delegatee] = nCheckpoints + 1; } emit DelegateVotesChanged(delegatee, oldVotes, newVotes); } function safe32(uint256 n, string memory errorMessage) internal pure returns (uint32) { require(n < 2**32, errorMessage); return uint32(n); } function safe96(uint256 n, string memory errorMessage) internal pure returns (uint96) { require(n < 2**96, errorMessage); return uint96(n); } function add96( uint96 a, uint96 b, string memory errorMessage ) internal pure returns (uint96) { uint96 c = a + b; require(c >= a, errorMessage); return c; } function sub96( uint96 a, uint96 b, string memory errorMessage ) internal pure returns (uint96) { require(b <= a, errorMessage); return a - b; } function getChainId() internal view returns (uint256) { uint256 chainId; assembly { chainId := chainid() } return chainId; } } // SPDX-License-Identifier: GPL-3.0 /// @title Interface for NounsDescriptor /********************************* * ░░░░░░░░░░░░░░░░░░░░░░░░░░░░░ * * ░░░░░░░░░░░░░░░░░░░░░░░░░░░░░ * * ░░░░░░█████████░░█████████░░░ * * ░░░░░░██░░░████░░██░░░████░░░ * * ░░██████░░░████████░░░████░░░ * * ░░██░░██░░░████░░██░░░████░░░ * * ░░██░░██░░░████░░██░░░████░░░ * * ░░░░░░█████████░░█████████░░░ * * ░░░░░░░░░░░░░░░░░░░░░░░░░░░░░ * * ░░░░░░░░░░░░░░░░░░░░░░░░░░░░░ * *********************************/ pragma solidity ^0.8.6; import { INounsSeeder } from './INounsSeeder.sol'; interface INounsDescriptor { event PartsLocked(); event DataURIToggled(bool enabled); event BaseURIUpdated(string baseURI); function arePartsLocked() external returns (bool); function isDataURIEnabled() external returns (bool); function baseURI() external returns (string memory); function palettes(uint8 paletteIndex, uint256 colorIndex) external view returns (string memory); function backgrounds(uint256 index) external view returns (string memory); function bodies(uint256 index) external view returns (bytes memory); function accessories(uint256 index) external view returns (bytes memory); function heads(uint256 index) external view returns (bytes memory); function glasses(uint256 index) external view returns (bytes memory); function backgroundCount() external view returns (uint256); function bodyCount() external view returns (uint256); function accessoryCount() external view returns (uint256); function headCount() external view returns (uint256); function glassesCount() external view returns (uint256); function addManyColorsToPalette(uint8 paletteIndex, string[] calldata newColors) external; function addManyBackgrounds(string[] calldata backgrounds) external; function addManyBodies(bytes[] calldata bodies) external; function addManyAccessories(bytes[] calldata accessories) external; function addManyHeads(bytes[] calldata heads) external; function addManyGlasses(bytes[] calldata glasses) external; function addColorToPalette(uint8 paletteIndex, string calldata color) external; function addBackground(string calldata background) external; function addBody(bytes calldata body) external; function addAccessory(bytes calldata accessory) external; function addHead(bytes calldata head) external; function addGlasses(bytes calldata glasses) external; function lockParts() external; function toggleDataURIEnabled() external; function setBaseURI(string calldata baseURI) external; function tokenURI(uint256 tokenId, INounsSeeder.Seed memory seed) external view returns (string memory); function dataURI(uint256 tokenId, INounsSeeder.Seed memory seed) external view returns (string memory); function genericDataURI( string calldata name, string calldata description, INounsSeeder.Seed memory seed ) external view returns (string memory); function generateSVGImage(INounsSeeder.Seed memory seed) external view returns (string memory); } // SPDX-License-Identifier: GPL-3.0 /// @title Interface for NounsSeeder /********************************* * ░░░░░░░░░░░░░░░░░░░░░░░░░░░░░ * * ░░░░░░░░░░░░░░░░░░░░░░░░░░░░░ * * ░░░░░░█████████░░█████████░░░ * * ░░░░░░██░░░████░░██░░░████░░░ * * ░░██████░░░████████░░░████░░░ * * ░░██░░██░░░████░░██░░░████░░░ * * ░░██░░██░░░████░░██░░░████░░░ * * ░░░░░░█████████░░█████████░░░ * * ░░░░░░░░░░░░░░░░░░░░░░░░░░░░░ * * ░░░░░░░░░░░░░░░░░░░░░░░░░░░░░ * *********************************/ pragma solidity ^0.8.6; import { INounsDescriptor } from './INounsDescriptor.sol'; interface INounsSeeder { struct Seed { uint48 background; uint48 body; uint48 accessory; uint48 head; uint48 glasses; } function generateSeed(uint256 nounId, INounsDescriptor descriptor) external view returns (Seed memory); } // SPDX-License-Identifier: GPL-3.0 /// @title Interface for NounsToken /********************************* * ░░░░░░░░░░░░░░░░░░░░░░░░░░░░░ * * ░░░░░░░░░░░░░░░░░░░░░░░░░░░░░ * * ░░░░░░█████████░░█████████░░░ * * ░░░░░░██░░░████░░██░░░████░░░ * * ░░██████░░░████████░░░████░░░ * * ░░██░░██░░░████░░██░░░████░░░ * * ░░██░░██░░░████░░██░░░████░░░ * * ░░░░░░█████████░░█████████░░░ * * ░░░░░░░░░░░░░░░░░░░░░░░░░░░░░ * * ░░░░░░░░░░░░░░░░░░░░░░░░░░░░░ * *********************************/ pragma solidity ^0.8.6; import { IERC721 } from '@openzeppelin/contracts/token/ERC721/IERC721.sol'; import { INounsDescriptor } from './INounsDescriptor.sol'; import { INounsSeeder } from './INounsSeeder.sol'; interface INounsToken is IERC721 { event NounCreated(uint256 indexed tokenId, INounsSeeder.Seed seed); event NounBurned(uint256 indexed tokenId); event LilNoundersDAOUpdated(address lilnoundersDAO); event NounsDAOUpdated(address nounsDAO); event MinterUpdated(address minter); event MinterLocked(); event DescriptorUpdated(INounsDescriptor descriptor); event DescriptorLocked(); event SeederUpdated(INounsSeeder seeder); event SeederLocked(); function mint() external returns (uint256); function burn(uint256 tokenId) external; function dataURI(uint256 tokenId) external returns (string memory); function setLilNoundersDAO(address lilnoundersDAO) external; function setNounsDAO(address nounsDAO) external; function setMinter(address minter) external; function lockMinter() external; function setDescriptor(INounsDescriptor descriptor) external; function lockDescriptor() external; function setSeeder(INounsSeeder seeder) external; function lockSeeder() external; } // SPDX-License-Identifier: MIT /// @title ERC721 Token Implementation /********************************* * ░░░░░░░░░░░░░░░░░░░░░░░░░░░░░ * * ░░░░░░░░░░░░░░░░░░░░░░░░░░░░░ * * ░░░░░░█████████░░█████████░░░ * * ░░░░░░██░░░████░░██░░░████░░░ * * ░░██████░░░████████░░░████░░░ * * ░░██░░██░░░████░░██░░░████░░░ * * ░░██░░██░░░████░░██░░░████░░░ * * ░░░░░░█████████░░█████████░░░ * * ░░░░░░░░░░░░░░░░░░░░░░░░░░░░░ * * ░░░░░░░░░░░░░░░░░░░░░░░░░░░░░ * *********************************/ // LICENSE // ERC721.sol modifies OpenZeppelin's ERC721.sol: // https://github.com/OpenZeppelin/openzeppelin-contracts/blob/6618f9f18424ade44116d0221719f4c93be6a078/contracts/token/ERC721/ERC721.sol // // ERC721.sol source code copyright OpenZeppelin licensed under the MIT License. // With modifications by Nounders DAO. // // // MODIFICATIONS: // `_safeMint` and `_mint` contain an additional `creator` argument and // emit two `Transfer` logs, rather than one. The first log displays the // transfer (mint) from `address(0)` to the `creator`. The second displays the // transfer from the `creator` to the `to` address. This enables correct // attribution on various NFT marketplaces. pragma solidity ^0.8.6; import '@openzeppelin/contracts/token/ERC721/IERC721.sol'; import '@openzeppelin/contracts/token/ERC721/IERC721Receiver.sol'; import '@openzeppelin/contracts/token/ERC721/extensions/IERC721Metadata.sol'; import '@openzeppelin/contracts/utils/Address.sol'; import '@openzeppelin/contracts/utils/Context.sol'; import '@openzeppelin/contracts/utils/Strings.sol'; import '@openzeppelin/contracts/utils/introspection/ERC165.sol'; /** * @dev Implementation of https://eips.ethereum.org/EIPS/eip-721[ERC721] Non-Fungible Token Standard, including * the Metadata extension, but not including the Enumerable extension, which is available separately as * {ERC721Enumerable}. */ contract ERC721 is Context, ERC165, IERC721, IERC721Metadata { using Address for address; using Strings for uint256; // Token name string private _name; // Token symbol string private _symbol; // Mapping from token ID to owner address mapping(uint256 => address) private _owners; // Mapping owner address to token count mapping(address => uint256) private _balances; // Mapping from token ID to approved address mapping(uint256 => address) private _tokenApprovals; // Mapping from owner to operator approvals mapping(address => mapping(address => bool)) private _operatorApprovals; /** * @dev Initializes the contract by setting a `name` and a `symbol` to the token collection. */ constructor(string memory name_, string memory symbol_) { _name = name_; _symbol = symbol_; } /** * @dev See {IERC165-supportsInterface}. */ function supportsInterface(bytes4 interfaceId) public view virtual override(ERC165, IERC165) returns (bool) { return interfaceId == type(IERC721).interfaceId || interfaceId == type(IERC721Metadata).interfaceId || super.supportsInterface(interfaceId); } /** * @dev See {IERC721-balanceOf}. */ function balanceOf(address owner) public view virtual override returns (uint256) { require(owner != address(0), 'ERC721: balance query for the zero address'); return _balances[owner]; } /** * @dev See {IERC721-ownerOf}. */ function ownerOf(uint256 tokenId) public view virtual override returns (address) { address owner = _owners[tokenId]; require(owner != address(0), 'ERC721: owner query for nonexistent token'); return owner; } /** * @dev See {IERC721Metadata-name}. */ function name() public view virtual override returns (string memory) { return _name; } /** * @dev See {IERC721Metadata-symbol}. */ function symbol() public view virtual override returns (string memory) { return _symbol; } /** * @dev See {IERC721Metadata-tokenURI}. */ function tokenURI(uint256 tokenId) public view virtual override returns (string memory) { require(_exists(tokenId), 'ERC721Metadata: URI query for nonexistent token'); string memory baseURI = _baseURI(); return bytes(baseURI).length > 0 ? string(abi.encodePacked(baseURI, tokenId.toString())) : ''; } /** * @dev Base URI for computing {tokenURI}. If set, the resulting URI for each * token will be the concatenation of the `baseURI` and the `tokenId`. Empty * by default, can be overriden in child contracts. */ function _baseURI() internal view virtual returns (string memory) { return ''; } /** * @dev See {IERC721-approve}. */ function approve(address to, uint256 tokenId) public virtual override { address owner = ERC721.ownerOf(tokenId); require(to != owner, 'ERC721: approval to current owner'); require( _msgSender() == owner || isApprovedForAll(owner, _msgSender()), 'ERC721: approve caller is not owner nor approved for all' ); _approve(to, tokenId); } /** * @dev See {IERC721-getApproved}. */ function getApproved(uint256 tokenId) public view virtual override returns (address) { require(_exists(tokenId), 'ERC721: approved query for nonexistent token'); return _tokenApprovals[tokenId]; } /** * @dev See {IERC721-setApprovalForAll}. */ function setApprovalForAll(address operator, bool approved) public virtual override { require(operator != _msgSender(), 'ERC721: approve to caller'); _operatorApprovals[_msgSender()][operator] = approved; emit ApprovalForAll(_msgSender(), operator, approved); } /** * @dev See {IERC721-isApprovedForAll}. */ function isApprovedForAll(address owner, address operator) public view virtual override returns (bool) { return _operatorApprovals[owner][operator]; } /** * @dev See {IERC721-transferFrom}. */ function transferFrom( address from, address to, uint256 tokenId ) public virtual override { //solhint-disable-next-line max-line-length require(_isApprovedOrOwner(_msgSender(), tokenId), 'ERC721: transfer caller is not owner nor approved'); _transfer(from, to, tokenId); } /** * @dev See {IERC721-safeTransferFrom}. */ function safeTransferFrom( address from, address to, uint256 tokenId ) public virtual override { safeTransferFrom(from, to, tokenId, ''); } /** * @dev See {IERC721-safeTransferFrom}. */ function safeTransferFrom( address from, address to, uint256 tokenId, bytes memory _data ) public virtual override { require(_isApprovedOrOwner(_msgSender(), tokenId), 'ERC721: transfer caller is not owner nor approved'); _safeTransfer(from, to, tokenId, _data); } /** * @dev Safely transfers `tokenId` token from `from` to `to`, checking first that contract recipients * are aware of the ERC721 protocol to prevent tokens from being forever locked. * * `_data` is additional data, it has no specified format and it is sent in call to `to`. * * This internal function is equivalent to {safeTransferFrom}, and can be used to e.g. * implement alternative mechanisms to perform token transfer, such as signature-based. * * Requirements: * * - `from` cannot be the zero address. * - `to` cannot be the zero address. * - `tokenId` token must exist and be owned by `from`. * - If `to` refers to a smart contract, it must implement {IERC721Receiver-onERC721Received}, which is called upon a safe transfer. * * Emits a {Transfer} event. */ function _safeTransfer( address from, address to, uint256 tokenId, bytes memory _data ) internal virtual { _transfer(from, to, tokenId); require(_checkOnERC721Received(from, to, tokenId, _data), 'ERC721: transfer to non ERC721Receiver implementer'); } /** * @dev Returns whether `tokenId` exists. * * Tokens can be managed by their owner or approved accounts via {approve} or {setApprovalForAll}. * * Tokens start existing when they are minted (`_mint`), * and stop existing when they are burned (`_burn`). */ function _exists(uint256 tokenId) internal view virtual returns (bool) { return _owners[tokenId] != address(0); } /** * @dev Returns whether `spender` is allowed to manage `tokenId`. * * Requirements: * * - `tokenId` must exist. */ function _isApprovedOrOwner(address spender, uint256 tokenId) internal view virtual returns (bool) { require(_exists(tokenId), 'ERC721: operator query for nonexistent token'); address owner = ERC721.ownerOf(tokenId); return (spender == owner || getApproved(tokenId) == spender || isApprovedForAll(owner, spender)); } /** * @dev Safely mints `tokenId`, transfers it to `to`, and emits two log events - * 1. Credits the `minter` with the mint. * 2. Shows transfer from the `minter` to `to`. * * Requirements: * * - `tokenId` must not exist. * - If `to` refers to a smart contract, it must implement {IERC721Receiver-onERC721Received}, which is called upon a safe transfer. * * Emits a {Transfer} event. */ function _safeMint( address creator, address to, uint256 tokenId ) internal virtual { _safeMint(creator, to, tokenId, ''); } /** * @dev Same as {xref-ERC721-_safeMint-address-uint256-}[`_safeMint`], with an additional `data` parameter which is * forwarded in {IERC721Receiver-onERC721Received} to contract recipients. */ function _safeMint( address creator, address to, uint256 tokenId, bytes memory _data ) internal virtual { _mint(creator, to, tokenId); require( _checkOnERC721Received(address(0), to, tokenId, _data), 'ERC721: transfer to non ERC721Receiver implementer' ); } /** * @dev Mints `tokenId`, transfers it to `to`, and emits two log events - * 1. Credits the `creator` with the mint. * 2. Shows transfer from the `creator` to `to`. * * WARNING: Usage of this method is discouraged, use {_safeMint} whenever possible * * Requirements: * * - `tokenId` must not exist. * - `to` cannot be the zero address. * * Emits a {Transfer} event. */ function _mint( address creator, address to, uint256 tokenId ) internal virtual { require(to != address(0), 'ERC721: mint to the zero address'); require(!_exists(tokenId), 'ERC721: token already minted'); _beforeTokenTransfer(address(0), to, tokenId); _balances[to] += 1; _owners[tokenId] = to; emit Transfer(address(0), creator, tokenId); emit Transfer(creator, to, tokenId); } /** * @dev Destroys `tokenId`. * The approval is cleared when the token is burned. * * Requirements: * * - `tokenId` must exist. * * Emits a {Transfer} event. */ function _burn(uint256 tokenId) internal virtual { address owner = ERC721.ownerOf(tokenId); _beforeTokenTransfer(owner, address(0), tokenId); // Clear approvals _approve(address(0), tokenId); _balances[owner] -= 1; delete _owners[tokenId]; emit Transfer(owner, address(0), tokenId); } /** * @dev Transfers `tokenId` from `from` to `to`. * As opposed to {transferFrom}, this imposes no restrictions on msg.sender. * * Requirements: * * - `to` cannot be the zero address. * - `tokenId` token must be owned by `from`. * * Emits a {Transfer} event. */ function _transfer( address from, address to, uint256 tokenId ) internal virtual { require(ERC721.ownerOf(tokenId) == from, 'ERC721: transfer of token that is not own'); require(to != address(0), 'ERC721: transfer to the zero address'); _beforeTokenTransfer(from, to, tokenId); // Clear approvals from the previous owner _approve(address(0), tokenId); _balances[from] -= 1; _balances[to] += 1; _owners[tokenId] = to; emit Transfer(from, to, tokenId); } /** * @dev Approve `to` to operate on `tokenId` * * Emits a {Approval} event. */ function _approve(address to, uint256 tokenId) internal virtual { _tokenApprovals[tokenId] = to; emit Approval(ERC721.ownerOf(tokenId), to, tokenId); } /** * @dev Internal function to invoke {IERC721Receiver-onERC721Received} on a target address. * The call is not executed if the target address is not a contract. * * @param from address representing the previous owner of the given token ID * @param to target address that will receive the tokens * @param tokenId uint256 ID of the token to be transferred * @param _data bytes optional data to send along with the call * @return bool whether the call correctly returned the expected magic value */ function _checkOnERC721Received( address from, address to, uint256 tokenId, bytes memory _data ) private returns (bool) { if (to.isContract()) { try IERC721Receiver(to).onERC721Received(_msgSender(), from, tokenId, _data) returns (bytes4 retval) { return retval == IERC721Receiver(to).onERC721Received.selector; } catch (bytes memory reason) { if (reason.length == 0) { revert('ERC721: transfer to non ERC721Receiver implementer'); } else { assembly { revert(add(32, reason), mload(reason)) } } } } else { return true; } } /** * @dev Hook that is called before any token transfer. This includes minting * and burning. * * Calling conditions: * * - When `from` and `to` are both non-zero, ``from``'s `tokenId` will be * transferred to `to`. * - When `from` is zero, `tokenId` will be minted for `to`. * - When `to` is zero, ``from``'s `tokenId` will be burned. * - `from` and `to` are never both zero. * * To learn more about hooks, head to xref:ROOT:extending-contracts.adoc#using-hooks[Using Hooks]. */ function _beforeTokenTransfer( address from, address to, uint256 tokenId ) internal virtual {} } // SPDX-License-Identifier: MIT // OpenZeppelin Contracts v4.4.0 (token/ERC721/IERC721.sol) pragma solidity ^0.8.0; import "../../utils/introspection/IERC165.sol"; /** * @dev Required interface of an ERC721 compliant contract. */ interface IERC721 is IERC165 { /** * @dev Emitted when `tokenId` token is transferred from `from` to `to`. */ event Transfer(address indexed from, address indexed to, uint256 indexed tokenId); /** * @dev Emitted when `owner` enables `approved` to manage the `tokenId` token. */ event Approval(address indexed owner, address indexed approved, uint256 indexed tokenId); /** * @dev Emitted when `owner` enables or disables (`approved`) `operator` to manage all of its assets. */ event ApprovalForAll(address indexed owner, address indexed operator, bool approved); /** * @dev Returns the number of tokens in ``owner``'s account. */ function balanceOf(address owner) external view returns (uint256 balance); /** * @dev Returns the owner of the `tokenId` token. * * Requirements: * * - `tokenId` must exist. */ function ownerOf(uint256 tokenId) external view returns (address owner); /** * @dev Safely transfers `tokenId` token from `from` to `to`, checking first that contract recipients * are aware of the ERC721 protocol to prevent tokens from being forever locked. * * Requirements: * * - `from` cannot be the zero address. * - `to` cannot be the zero address. * - `tokenId` token must exist and be owned by `from`. * - If the caller is not `from`, it must be have been allowed to move this token by either {approve} or {setApprovalForAll}. * - If `to` refers to a smart contract, it must implement {IERC721Receiver-onERC721Received}, which is called upon a safe transfer. * * Emits a {Transfer} event. */ function safeTransferFrom( address from, address to, uint256 tokenId ) external; /** * @dev Transfers `tokenId` token from `from` to `to`. * * WARNING: Usage of this method is discouraged, use {safeTransferFrom} whenever possible. * * Requirements: * * - `from` cannot be the zero address. * - `to` cannot be the zero address. * - `tokenId` token must be owned by `from`. * - If the caller is not `from`, it must be approved to move this token by either {approve} or {setApprovalForAll}. * * Emits a {Transfer} event. */ function transferFrom( address from, address to, uint256 tokenId ) external; /** * @dev Gives permission to `to` to transfer `tokenId` token to another account. * The approval is cleared when the token is transferred. * * Only a single account can be approved at a time, so approving the zero address clears previous approvals. * * Requirements: * * - The caller must own the token or be an approved operator. * - `tokenId` must exist. * * Emits an {Approval} event. */ function approve(address to, uint256 tokenId) external; /** * @dev Returns the account approved for `tokenId` token. * * Requirements: * * - `tokenId` must exist. */ function getApproved(uint256 tokenId) external view returns (address operator); /** * @dev Approve or remove `operator` as an operator for the caller. * Operators can call {transferFrom} or {safeTransferFrom} for any token owned by the caller. * * Requirements: * * - The `operator` cannot be the caller. * * Emits an {ApprovalForAll} event. */ function setApprovalForAll(address operator, bool _approved) external; /** * @dev Returns if the `operator` is allowed to manage all of the assets of `owner`. * * See {setApprovalForAll} */ function isApprovedForAll(address owner, address operator) external view returns (bool); /** * @dev Safely transfers `tokenId` token from `from` to `to`. * * Requirements: * * - `from` cannot be the zero address. * - `to` cannot be the zero address. * - `tokenId` token must exist and be owned by `from`. * - If the caller is not `from`, it must be approved to move this token by either {approve} or {setApprovalForAll}. * - If `to` refers to a smart contract, it must implement {IERC721Receiver-onERC721Received}, which is called upon a safe transfer. * * Emits a {Transfer} event. */ function safeTransferFrom( address from, address to, uint256 tokenId, bytes calldata data ) external; } // SPDX-License-Identifier: MIT pragma solidity ^0.8.6; interface IProxyRegistry { function proxies(address) external view returns (address); } // SPDX-License-Identifier: MIT // OpenZeppelin Contracts v4.4.0 (utils/Context.sol) pragma solidity ^0.8.0; /** * @dev Provides information about the current execution context, including the * sender of the transaction and its data. While these are generally available * via msg.sender and msg.data, they should not be accessed in such a direct * manner, since when dealing with meta-transactions the account sending and * paying for execution may not be the actual sender (as far as an application * is concerned). * * This contract is only required for intermediate, library-like contracts. */ abstract contract Context { function _msgSender() internal view virtual returns (address) { return msg.sender; } function _msgData() internal view virtual returns (bytes calldata) { return msg.data; } } // SPDX-License-Identifier: MIT /// @title ERC721 Enumerable Extension /********************************* * ░░░░░░░░░░░░░░░░░░░░░░░░░░░░░ * * ░░░░░░░░░░░░░░░░░░░░░░░░░░░░░ * * ░░░░░░█████████░░█████████░░░ * * ░░░░░░██░░░████░░██░░░████░░░ * * ░░██████░░░████████░░░████░░░ * * ░░██░░██░░░████░░██░░░████░░░ * * ░░██░░██░░░████░░██░░░████░░░ * * ░░░░░░█████████░░█████████░░░ * * ░░░░░░░░░░░░░░░░░░░░░░░░░░░░░ * * ░░░░░░░░░░░░░░░░░░░░░░░░░░░░░ * *********************************/ // LICENSE // ERC721.sol modifies OpenZeppelin's ERC721Enumerable.sol: // https://github.com/OpenZeppelin/openzeppelin-contracts/blob/6618f9f18424ade44116d0221719f4c93be6a078/contracts/token/ERC721/extensions/ERC721Enumerable.sol // // ERC721Enumerable.sol source code copyright OpenZeppelin licensed under the MIT License. // With modifications by Nounders DAO. // // MODIFICATIONS: // Consumes modified `ERC721` contract. See notes in `ERC721.sol`. pragma solidity ^0.8.0; import './ERC721.sol'; import '@openzeppelin/contracts/token/ERC721/extensions/IERC721Enumerable.sol'; /** * @dev This implements an optional extension of {ERC721} defined in the EIP that adds * enumerability of all the token ids in the contract as well as all token ids owned by each * account. */ abstract contract ERC721Enumerable is ERC721, IERC721Enumerable { // Mapping from owner to list of owned token IDs mapping(address => mapping(uint256 => uint256)) private _ownedTokens; // Mapping from token ID to index of the owner tokens list mapping(uint256 => uint256) private _ownedTokensIndex; // Array with all token ids, used for enumeration uint256[] private _allTokens; // Mapping from token id to position in the allTokens array mapping(uint256 => uint256) private _allTokensIndex; /** * @dev See {IERC165-supportsInterface}. */ function supportsInterface(bytes4 interfaceId) public view virtual override(IERC165, ERC721) returns (bool) { return interfaceId == type(IERC721Enumerable).interfaceId || super.supportsInterface(interfaceId); } /** * @dev See {IERC721Enumerable-tokenOfOwnerByIndex}. */ function tokenOfOwnerByIndex(address owner, uint256 index) public view virtual override returns (uint256) { require(index < ERC721.balanceOf(owner), 'ERC721Enumerable: owner index out of bounds'); return _ownedTokens[owner][index]; } /** * @dev See {IERC721Enumerable-totalSupply}. */ function totalSupply() public view virtual override returns (uint256) { return _allTokens.length; } /** * @dev See {IERC721Enumerable-tokenByIndex}. */ function tokenByIndex(uint256 index) public view virtual override returns (uint256) { require(index < ERC721Enumerable.totalSupply(), 'ERC721Enumerable: global index out of bounds'); return _allTokens[index]; } /** * @dev Hook that is called before any token transfer. This includes minting * and burning. * * Calling conditions: * * - When `from` and `to` are both non-zero, ``from``'s `tokenId` will be * transferred to `to`. * - When `from` is zero, `tokenId` will be minted for `to`. * - When `to` is zero, ``from``'s `tokenId` will be burned. * - `from` cannot be the zero address. * - `to` cannot be the zero address. * * To learn more about hooks, head to xref:ROOT:extending-contracts.adoc#using-hooks[Using Hooks]. */ function _beforeTokenTransfer( address from, address to, uint256 tokenId ) internal virtual override { super._beforeTokenTransfer(from, to, tokenId); if (from == address(0)) { _addTokenToAllTokensEnumeration(tokenId); } else if (from != to) { _removeTokenFromOwnerEnumeration(from, tokenId); } if (to == address(0)) { _removeTokenFromAllTokensEnumeration(tokenId); } else if (to != from) { _addTokenToOwnerEnumeration(to, tokenId); } } /** * @dev Private function to add a token to this extension's ownership-tracking data structures. * @param to address representing the new owner of the given token ID * @param tokenId uint256 ID of the token to be added to the tokens list of the given address */ function _addTokenToOwnerEnumeration(address to, uint256 tokenId) private { uint256 length = ERC721.balanceOf(to); _ownedTokens[to][length] = tokenId; _ownedTokensIndex[tokenId] = length; } /** * @dev Private function to add a token to this extension's token tracking data structures. * @param tokenId uint256 ID of the token to be added to the tokens list */ function _addTokenToAllTokensEnumeration(uint256 tokenId) private { _allTokensIndex[tokenId] = _allTokens.length; _allTokens.push(tokenId); } /** * @dev Private function to remove a token from this extension's ownership-tracking data structures. Note that * while the token is not assigned a new owner, the `_ownedTokensIndex` mapping is _not_ updated: this allows for * gas optimizations e.g. when performing a transfer operation (avoiding double writes). * This has O(1) time complexity, but alters the order of the _ownedTokens array. * @param from address representing the previous owner of the given token ID * @param tokenId uint256 ID of the token to be removed from the tokens list of the given address */ function _removeTokenFromOwnerEnumeration(address from, uint256 tokenId) private { // To prevent a gap in from's tokens array, we store the last token in the index of the token to delete, and // then delete the last slot (swap and pop). uint256 lastTokenIndex = ERC721.balanceOf(from) - 1; uint256 tokenIndex = _ownedTokensIndex[tokenId]; // When the token to delete is the last token, the swap operation is unnecessary if (tokenIndex != lastTokenIndex) { uint256 lastTokenId = _ownedTokens[from][lastTokenIndex]; _ownedTokens[from][tokenIndex] = lastTokenId; // Move the last token to the slot of the to-delete token _ownedTokensIndex[lastTokenId] = tokenIndex; // Update the moved token's index } // This also deletes the contents at the last position of the array delete _ownedTokensIndex[tokenId]; delete _ownedTokens[from][lastTokenIndex]; } /** * @dev Private function to remove a token from this extension's token tracking data structures. * This has O(1) time complexity, but alters the order of the _allTokens array. * @param tokenId uint256 ID of the token to be removed from the tokens list */ function _removeTokenFromAllTokensEnumeration(uint256 tokenId) private { // To prevent a gap in the tokens array, we store the last token in the index of the token to delete, and // then delete the last slot (swap and pop). uint256 lastTokenIndex = _allTokens.length - 1; uint256 tokenIndex = _allTokensIndex[tokenId]; // When the token to delete is the last token, the swap operation is unnecessary. However, since this occurs so // rarely (when the last minted token is burnt) that we still do the swap here to avoid the gas cost of adding // an 'if' statement (like in _removeTokenFromOwnerEnumeration) uint256 lastTokenId = _allTokens[lastTokenIndex]; _allTokens[tokenIndex] = lastTokenId; // Move the last token to the slot of the to-delete token _allTokensIndex[lastTokenId] = tokenIndex; // Update the moved token's index // This also deletes the contents at the last position of the array delete _allTokensIndex[tokenId]; _allTokens.pop(); } } // SPDX-License-Identifier: MIT // OpenZeppelin Contracts v4.4.0 (token/ERC721/extensions/IERC721Enumerable.sol) pragma solidity ^0.8.0; import "../IERC721.sol"; /** * @title ERC-721 Non-Fungible Token Standard, optional enumeration extension * @dev See https://eips.ethereum.org/EIPS/eip-721 */ interface IERC721Enumerable is IERC721 { /** * @dev Returns the total amount of tokens stored by the contract. */ function totalSupply() external view returns (uint256); /** * @dev Returns a token ID owned by `owner` at a given `index` of its token list. * Use along with {balanceOf} to enumerate all of ``owner``'s tokens. */ function tokenOfOwnerByIndex(address owner, uint256 index) external view returns (uint256 tokenId); /** * @dev Returns a token ID at a given `index` of all the tokens stored by the contract. * Use along with {totalSupply} to enumerate all tokens. */ function tokenByIndex(uint256 index) external view returns (uint256); } // SPDX-License-Identifier: MIT // OpenZeppelin Contracts v4.4.0 (token/ERC721/IERC721Receiver.sol) pragma solidity ^0.8.0; /** * @title ERC721 token receiver interface * @dev Interface for any contract that wants to support safeTransfers * from ERC721 asset contracts. */ interface IERC721Receiver { /** * @dev Whenever an {IERC721} `tokenId` token is transferred to this contract via {IERC721-safeTransferFrom} * by `operator` from `from`, this function is called. * * It must return its Solidity selector to confirm the token transfer. * If any other value is returned or the interface is not implemented by the recipient, the transfer will be reverted. * * The selector can be obtained in Solidity with `IERC721.onERC721Received.selector`. */ function onERC721Received( address operator, address from, uint256 tokenId, bytes calldata data ) external returns (bytes4); } // SPDX-License-Identifier: MIT // OpenZeppelin Contracts v4.4.0 (token/ERC721/extensions/IERC721Metadata.sol) pragma solidity ^0.8.0; import "../IERC721.sol"; /** * @title ERC-721 Non-Fungible Token Standard, optional metadata extension * @dev See https://eips.ethereum.org/EIPS/eip-721 */ interface IERC721Metadata is IERC721 { /** * @dev Returns the token collection name. */ function name() external view returns (string memory); /** * @dev Returns the token collection symbol. */ function symbol() external view returns (string memory); /** * @dev Returns the Uniform Resource Identifier (URI) for `tokenId` token. */ function tokenURI(uint256 tokenId) external view returns (string memory); } // SPDX-License-Identifier: MIT // OpenZeppelin Contracts v4.4.0 (utils/Address.sol) pragma solidity ^0.8.0; /** * @dev Collection of functions related to the address type */ library Address { /** * @dev Returns true if `account` is a contract. * * [IMPORTANT] * ==== * It is unsafe to assume that an address for which this function returns * false is an externally-owned account (EOA) and not a contract. * * Among others, `isContract` will return false for the following * types of addresses: * * - an externally-owned account * - a contract in construction * - an address where a contract will be created * - an address where a contract lived, but was destroyed * ==== */ function isContract(address account) internal view returns (bool) { // This method relies on extcodesize, which returns 0 for contracts in // construction, since the code is only stored at the end of the // constructor execution. uint256 size; assembly { size := extcodesize(account) } return size > 0; } /** * @dev Replacement for Solidity's `transfer`: sends `amount` wei to * `recipient`, forwarding all available gas and reverting on errors. * * https://eips.ethereum.org/EIPS/eip-1884[EIP1884] increases the gas cost * of certain opcodes, possibly making contracts go over the 2300 gas limit * imposed by `transfer`, making them unable to receive funds via * `transfer`. {sendValue} removes this limitation. * * https://diligence.consensys.net/posts/2019/09/stop-using-soliditys-transfer-now/[Learn more]. * * IMPORTANT: because control is transferred to `recipient`, care must be * taken to not create reentrancy vulnerabilities. Consider using * {ReentrancyGuard} or the * https://solidity.readthedocs.io/en/v0.5.11/security-considerations.html#use-the-checks-effects-interactions-pattern[checks-effects-interactions pattern]. */ function sendValue(address payable recipient, uint256 amount) internal { require(address(this).balance >= amount, "Address: insufficient balance"); (bool success, ) = recipient.call{value: amount}(""); require(success, "Address: unable to send value, recipient may have reverted"); } /** * @dev Performs a Solidity function call using a low level `call`. A * plain `call` is an unsafe replacement for a function call: use this * function instead. * * If `target` reverts with a revert reason, it is bubbled up by this * function (like regular Solidity function calls). * * Returns the raw returned data. To convert to the expected return value, * use https://solidity.readthedocs.io/en/latest/units-and-global-variables.html?highlight=abi.decode#abi-encoding-and-decoding-functions[`abi.decode`]. * * Requirements: * * - `target` must be a contract. * - calling `target` with `data` must not revert. * * _Available since v3.1._ */ function functionCall(address target, bytes memory data) internal returns (bytes memory) { return functionCall(target, data, "Address: low-level call failed"); } /** * @dev Same as {xref-Address-functionCall-address-bytes-}[`functionCall`], but with * `errorMessage` as a fallback revert reason when `target` reverts. * * _Available since v3.1._ */ function functionCall( address target, bytes memory data, string memory errorMessage ) internal returns (bytes memory) { return functionCallWithValue(target, data, 0, errorMessage); } /** * @dev Same as {xref-Address-functionCall-address-bytes-}[`functionCall`], * but also transferring `value` wei to `target`. * * Requirements: * * - the calling contract must have an ETH balance of at least `value`. * - the called Solidity function must be `payable`. * * _Available since v3.1._ */ function functionCallWithValue( address target, bytes memory data, uint256 value ) internal returns (bytes memory) { return functionCallWithValue(target, data, value, "Address: low-level call with value failed"); } /** * @dev Same as {xref-Address-functionCallWithValue-address-bytes-uint256-}[`functionCallWithValue`], but * with `errorMessage` as a fallback revert reason when `target` reverts. * * _Available since v3.1._ */ function functionCallWithValue( address target, bytes memory data, uint256 value, string memory errorMessage ) internal returns (bytes memory) { require(address(this).balance >= value, "Address: insufficient balance for call"); require(isContract(target), "Address: call to non-contract"); (bool success, bytes memory returndata) = target.call{value: value}(data); return verifyCallResult(success, returndata, errorMessage); } /** * @dev Same as {xref-Address-functionCall-address-bytes-}[`functionCall`], * but performing a static call. * * _Available since v3.3._ */ function functionStaticCall(address target, bytes memory data) internal view returns (bytes memory) { return functionStaticCall(target, data, "Address: low-level static call failed"); } /** * @dev Same as {xref-Address-functionCall-address-bytes-string-}[`functionCall`], * but performing a static call. * * _Available since v3.3._ */ function functionStaticCall( address target, bytes memory data, string memory errorMessage ) internal view returns (bytes memory) { require(isContract(target), "Address: static call to non-contract"); (bool success, bytes memory returndata) = target.staticcall(data); return verifyCallResult(success, returndata, errorMessage); } /** * @dev Same as {xref-Address-functionCall-address-bytes-}[`functionCall`], * but performing a delegate call. * * _Available since v3.4._ */ function functionDelegateCall(address target, bytes memory data) internal returns (bytes memory) { return functionDelegateCall(target, data, "Address: low-level delegate call failed"); } /** * @dev Same as {xref-Address-functionCall-address-bytes-string-}[`functionCall`], * but performing a delegate call. * * _Available since v3.4._ */ function functionDelegateCall( address target, bytes memory data, string memory errorMessage ) internal returns (bytes memory) { require(isContract(target), "Address: delegate call to non-contract"); (bool success, bytes memory returndata) = target.delegatecall(data); return verifyCallResult(success, returndata, errorMessage); } /** * @dev Tool to verifies that a low level call was successful, and revert if it wasn't, either by bubbling the * revert reason using the provided one. * * _Available since v4.3._ */ function verifyCallResult( bool success, bytes memory returndata, string memory errorMessage ) internal pure returns (bytes memory) { if (success) { return returndata; } else { // Look for revert reason and bubble it up if present if (returndata.length > 0) { // The easiest way to bubble the revert reason is using memory via assembly assembly { let returndata_size := mload(returndata) revert(add(32, returndata), returndata_size) } } else { revert(errorMessage); } } } } // SPDX-License-Identifier: MIT // OpenZeppelin Contracts v4.4.0 (utils/Strings.sol) pragma solidity ^0.8.0; /** * @dev String operations. */ library Strings { bytes16 private constant _HEX_SYMBOLS = "0123456789abcdef"; /** * @dev Converts a `uint256` to its ASCII `string` decimal representation. */ function toString(uint256 value) internal pure returns (string memory) { // Inspired by OraclizeAPI's implementation - MIT licence // https://github.com/oraclize/ethereum-api/blob/b42146b063c7d6ee1358846c198246239e9360e8/oraclizeAPI_0.4.25.sol if (value == 0) { return "0"; } uint256 temp = value; uint256 digits; while (temp != 0) { digits++; temp /= 10; } bytes memory buffer = new bytes(digits); while (value != 0) { digits -= 1; buffer[digits] = bytes1(uint8(48 + uint256(value % 10))); value /= 10; } return string(buffer); } /** * @dev Converts a `uint256` to its ASCII `string` hexadecimal representation. */ function toHexString(uint256 value) internal pure returns (string memory) { if (value == 0) { return "0x00"; } uint256 temp = value; uint256 length = 0; while (temp != 0) { length++; temp >>= 8; } return toHexString(value, length); } /** * @dev Converts a `uint256` to its ASCII `string` hexadecimal representation with fixed length. */ function toHexString(uint256 value, uint256 length) internal pure returns (string memory) { bytes memory buffer = new bytes(2 * length + 2); buffer[0] = "0"; buffer[1] = "x"; for (uint256 i = 2 * length + 1; i > 1; --i) { buffer[i] = _HEX_SYMBOLS[value & 0xf]; value >>= 4; } require(value == 0, "Strings: hex length insufficient"); return string(buffer); } } // SPDX-License-Identifier: MIT // OpenZeppelin Contracts v4.4.0 (utils/introspection/ERC165.sol) pragma solidity ^0.8.0; import "./IERC165.sol"; /** * @dev Implementation of the {IERC165} interface. * * Contracts that want to implement ERC165 should inherit from this contract and override {supportsInterface} to check * for the additional interface id that will be supported. For example: * * ```solidity * function supportsInterface(bytes4 interfaceId) public view virtual override returns (bool) { * return interfaceId == type(MyInterface).interfaceId || super.supportsInterface(interfaceId); * } * ``` * * Alternatively, {ERC165Storage} provides an easier to use but more expensive implementation. */ abstract contract ERC165 is IERC165 { /** * @dev See {IERC165-supportsInterface}. */ function supportsInterface(bytes4 interfaceId) public view virtual override returns (bool) { return interfaceId == type(IERC165).interfaceId; } } // SPDX-License-Identifier: MIT // OpenZeppelin Contracts v4.4.0 (utils/introspection/IERC165.sol) pragma solidity ^0.8.0; /** * @dev Interface of the ERC165 standard, as defined in the * https://eips.ethereum.org/EIPS/eip-165[EIP]. * * Implementers can declare support of contract interfaces, which can then be * queried by others ({ERC165Checker}). * * For an implementation, see {ERC165}. */ interface IERC165 { /** * @dev Returns true if this contract implements the interface defined by * `interfaceId`. See the corresponding * https://eips.ethereum.org/EIPS/eip-165#how-interfaces-are-identified[EIP section] * to learn more about how these ids are created. * * This function call must use less than 30 000 gas. */ function supportsInterface(bytes4 interfaceId) external view returns (bool); }